I have same issue, but I have this versions:
“@web3auth/base”: “^4.2.4”,
“@web3auth/core”: “^4.3.2”,
“@web3auth/modal”: “^4.3.1”,
“@web3auth/openlogin-adapter”: “^4.2.4”,
And set this:
import { WALLET_ADAPTERS, CHAIN_NAMESPACES } from ‘@web3auth/base’;
import { Web3AuthCore } from ‘@web3auth/core’;
import { OpenloginAdapter } from ‘@web3auth/openlogin-adapter’;
import { getAuth0User } from ‘./auth0Api’;
import RPC from ‘./web3RPC’;
import Wallet from ‘…/models/Wallet’;
export const init = async (setWeb3auth, setProvider, setUser, setWallet, setAuth0User, setError, lastVisitedURL) => {
try {
const web3auth = new Web3AuthCore({
clientId: import.meta.env.VITE_APP_WEB_3_CLIENT_ID,
web3AuthNetwork: ‘cyan’,
chainConfig: {
chainNamespace: CHAIN_NAMESPACES.EIP155,
chainId: ‘0x5’,
},
});
const openloginAdapter = new OpenloginAdapter({
loginSettings: {
sessionTime: 604800,
},
adapterSettings: {
uxMode: 'redirect',
loginConfig: {
jwt: {
verifier: 'XXXXXX',
typeOfLogin: 'jwt',
clientId: 'XXXXXXX',
},
},
},
});
web3auth.configureAdapter(openloginAdapter);
setWeb3auth(web3auth);
openloginAdapter.setAdapterSettings({
redirectUrl: lastVisitedURL,
});
await web3auth.init();
const initWallet = Wallet;
if (web3auth.provider) {
setProvider(web3auth.provider);
const rpc = new RPC(web3auth.provider);
initWallet.address = await rpc.getAccount();
setWallet(initWallet);
}
const initUser = await web3auth.getUserInfo();
const auth0User = await getAuth0User(initUser);
// setUser({ ...initUser, name: auth0User.name, profileImage: auth0User.picture });
setUser(initUser);
setAuth0User(auth0User);
} catch (error) {
setError(error);
}
};
export const web3Login = async (web3auth, setProvider, setUser, setWallet, setAuth0User) => {
if (!web3auth) return;
const web3authProvider = await web3auth.connectTo(
WALLET_ADAPTERS.OPENLOGIN,
{
loginSettings: {
sessionTime: 259200,
},
loginProvider: ‘jwt’,
extraLoginOptions: {
verifierIdField: ‘sub’,
domain: import.meta.env.VITE_APP_AUTH0_LOGIN_URL,
},
},
);
setProvider(web3authProvider);
const initWallet = {};
const rpc = new RPC(web3authProvider);
initWallet.address = await rpc.getAccount();
setWallet(initWallet);
const initUser = await web3auth.getUserInfo();
setUser(initUser);
const auth0User = await getAuth0User(initUser);
setAuth0User(auth0User);
return { initWallet, initUser, auth0User };
};
export const web3Logout = async (web3auth, setProvider) => {
if (!web3auth) throw new Error(‘web3auth not initialized yet’);
await web3auth.logout();
setProvider(null);
};
But I have the error:
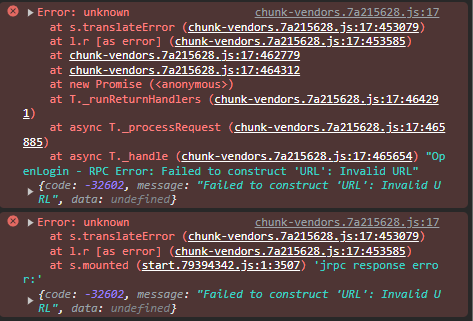