We are developing a dapp based on nextjs. We often encounter messages like this when logging in using web3auth’s openlogin.
At first I thought it was a problem with hosting on localhost, but I’ve encountered this error in production as well.
It usually resolved itself over time. Or it resolved for some unknown reason. (e.g. turning the computer off and on, killing all Chrome processes, or clearing browser caching)
Here’s what we’ve done so far to try and fix the error.
- Purchase and swap to a project in Mainnet environment
- Add hosted ip or localhost to whitelisted domains from the dashboard
- Reset browser cookies and localstorage
- secret browser
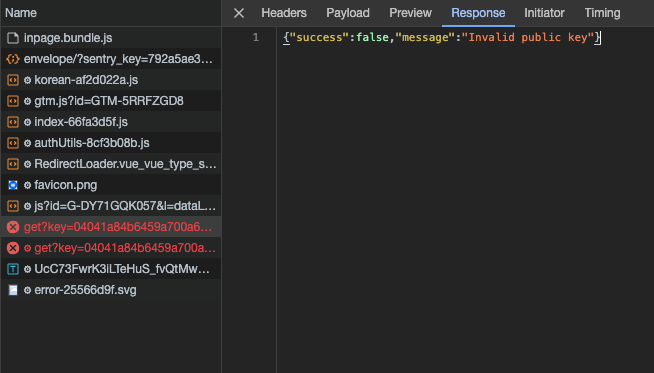
ERROR
Error: 404 cors
StartPageError: Invalid login session. Please restart the login process again.,
When i call the web3auth.connectTo function,i see these messages by taking a console snapshot of the https://auth.web3auth.io requested.
This error, which seems to be more common in development environments, has been causing me a lot of headaches lately. I can’t figure out what to do about it. we need your help,
-
SDK Version:
“@web3auth/base”: “^7.0.4”,
“@web3auth/modal”: “^7.0.4”,
“@web3auth/no-modal”: “^7.0.4”,
“@web3auth/openlogin-adapter”: “^7.0.4”,
“@web3auth/torus-wallet-connector-plugin”: “^7.0.4”, -
Platform:
Web -
Browser Console Screenshots:
-
Related to Custom Authentication? Please provide the following info too: (Optional)
- Verifier Name:
- JWKS Endpoint:
- Sample idToken(JWT)
Please provide the Web3Auth initialization and login code snippet below:
import React, {
createContext,
ReactNode,
useContext,
useEffect,
useMemo,
useState
} from 'react'
import { Web3AuthNoModal } from '@web3auth/no-modal'
import { CHAIN_NAMESPACES, IProvider, WALLET_ADAPTERS } from '@web3auth/base'
import Config from '~/config'
import { EthereumPrivateKeyProvider } from '@web3auth/ethereum-provider'
import {
OPENLOGIN_NETWORK,
OpenloginAdapter
} from '@web3auth/openlogin-adapter'
import { signIn, signOut, useSession } from 'next-auth/react'
import { InternalPath } from '~/constants/route'
import { ethers, Signer } from 'ethers'
import { v4 as uuidv4 } from 'uuid'
import { useRouter } from 'next/router'
import { LOGIN_PROVIDER_TYPE } from '@toruslabs/openlogin-utils/src/interfaces'
const Web3AuthContext = createContext<{
web3Auth?: Web3AuthNoModal
provider?: IProvider
signer?: Signer
logout?: () => void
connectSocialWallet?: (loginProvider: LOGIN_PROVIDER_TYPE) => void
}>({
web3Auth: null,
provider: null,
signer: null
})
const Web3AuthProvider = ({ children }: { children: ReactNode }) => {
const [web3Auth, setWeb3Auth] = useState<Web3AuthNoModal | null>(null)
const [provider, setProvider] = useState<IProvider | null>(null)
const [signer, setSigner] = useState<Signer | null>(null)
const { status } = useSession()
const { push } = useRouter()
const initializeWeb3Auth = async () => {
try {
const chainConfig = {
chainNamespace: CHAIN_NAMESPACES.EIP155,
chainId: '0x13881',
rpcTarget: Config.SERVER_RPC_URL,
displayName: 'Mumbai',
blockExplorer: 'https://mumbai.polygonscan.com',
ticker: 'MATIC',
tickerName: 'Matic'
}
const web3authInstance = new Web3AuthNoModal({
clientId: Config.WEB3_AUTH_CLIENT_ID,
chainConfig,
web3AuthNetwork: OPENLOGIN_NETWORK.SAPPHIRE_MAINNET,
sessionTime: Config.SESSION_TIME,
enableLogging: true
})
setWeb3Auth(web3authInstance)
const privateKeyProvider = new EthereumPrivateKeyProvider({
config: { chainConfig }
})
const openloginAdapter = new OpenloginAdapter({
privateKeyProvider,
adapterSettings: {
network: 'sapphire_mainnet',
uxMode: 'redirect',
loginConfig: {
google: {
verifier: 'google-dev',
clientId: Config.GOOGLE_CLIENT_ID,
typeOfLogin: 'google'
}
}
}
})
web3authInstance.configureAdapter(openloginAdapter)
await web3authInstance.init()
setProvider(web3authInstance.provider)
} catch (error) {
console.error(error)
}
}
const connectSocialWallet = async (loginProvider: LOGIN_PROVIDER_TYPE) => {
await web3Auth.connectTo(WALLET_ADAPTERS.OPENLOGIN, {
loginProvider,
mfaLevel: 'none'
})
}
const logout = async () => {
if (web3Auth?.connectedAdapterName) {
await web3Auth?.logout()
}
await signOut({ callbackUrl: InternalPath.HOME, redirect: true })
}
const value = useMemo(
() => ({
web3Auth,
provider,
signer,
logout,
connectSocialWallet
}),
[web3Auth, provider, signer, logout, connectSocialWallet]
)
return (
<Web3AuthContext.Provider value={value}>
{children}
</Web3AuthContext.Provider>
)
}
export const useWeb3AuthContext = () => useContext(Web3AuthContext)
export default Web3AuthProvider
I always called web3auth’s connectTo via the connectSocialWallet
function.