Whitelabel PnP React Native SDK
whiteLabel
For defining custom UI, branding, and translations for your brand app, you just need to define an
optional object called WhiteLabelData
.
This is a paid feature and the minimum pricing plan to use this SDK in a production environment is the Growth Plan. You can use this feature in Web3Auth Sapphire Devnet network for free.
Arguments
WhiteLabelData
- Table
- Interface
Parameter | Description |
---|---|
appName? | Display name for the app in the UI. |
logoLight? | App logo to be used in dark mode. It accepts url in string as a value. |
logoDark? | App logo to be used in light mode. It accepts url in string as a value. |
defaultLanguage? | Language which will be used by Web3Auth, app will use browser language if not specified. Default language is LANGUAGE_TYPE.en . Checkout LANGUAGE_TYPE for supported languages. |
mode? | Theme mode for the login modal. Choose between THEME_MODE_TYPE.auto , THEME_MODE_TYPE.light or THEME_MODE_TYPE.dark background modes. Default value is ThemeModes.auto . |
theme? | Used to customize the theme of the login modal. It accepts Record as a value. |
appUrl? | Url to be used in the Modal. It accepts url in string as a value. |
useLogoLoader? | Use logo loader. If logoDark and logoLight are null, the default Web3Auth logo will be used for the loader. Default value is false. |
tncLink | Terms and conditions link in Partial<Record<LANGUAGE_TYPE, string>> . |
privacyPolicy | Privacy policy link in Partial<Record<LANGUAGE_TYPE, string>> . |
export type WhiteLabelData = {
/**
* App name to display in the UI
*/
appName?: string;
/**
* App url
*/
appUrl?: string;
/**
* App logo to use in light mode
*/
logoLight?: string;
/**
* App logo to use in dark mode
*/
logoDark?: string;
/**
* language which will be used by web3auth. app will use browser language if not specified. if language is not supported it will use "en"
* en: english
* de: german
* ja: japanese
* ko: korean
* zh: mandarin
* es: spanish
* fr: french
* pt: portuguese
* nl: dutch
*
* @defaultValue en
*/
defaultLanguage?: LANGUAGE_TYPE;
/**
theme
*
* @defaultValue auto
*/
mode?: THEME_MODE_TYPE;
/**
* Use logo loader
*
* @defaultValue false
*/
useLogoLoader?: boolean;
/**
* Used to customize theme of the login modal with following options
* `'primary'` - To customize primary color of modal's content.
*/
theme?: {
primary?: string;
gray?: string;
red?: string;
green?: string;
success?: string;
warning?: string;
error?: string;
info?: string;
white?: string;
};
/**
* Language specific link for terms and conditions on torus-website. See (examples/vue-app) to configure
* e.g.
* tncLink: {
* en: "http://example.com/tnc/en",
* ja: "http://example.com/tnc/ja",
* }
*/
tncLink?: Partial<Record<LANGUAGE_TYPE, string>>;
/**
* Language specific link for privacy policy on torus-website. See (examples/vue-app) to configure
* e.g.
* privacyPolicy: {
* en: "http://example.com/tnc/en",
* ja: "http://example.com/tnc/ja",
* }
*/
privacyPolicy?: Partial<Record<LANGUAGE_TYPE, string>>;
};
name
The name of the application. This will be displayed in the key reconstruction page.
Standard screen without any change
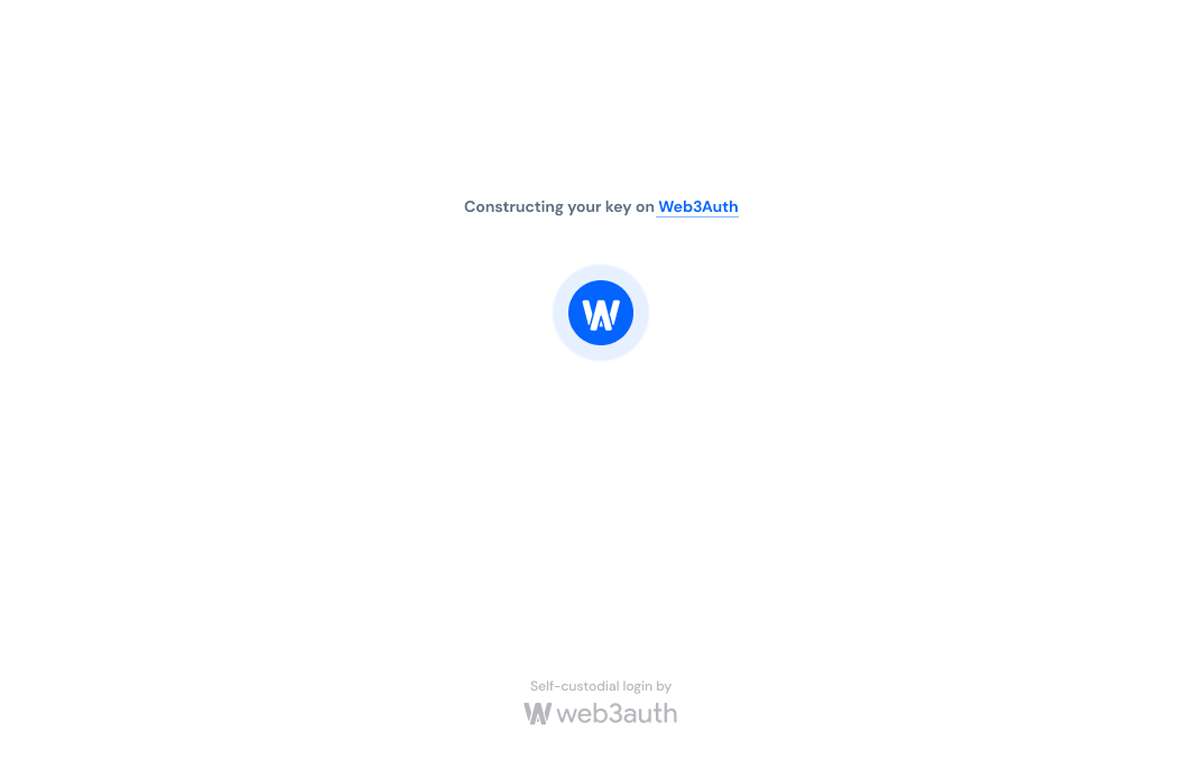
Name changed to Formidable Duo
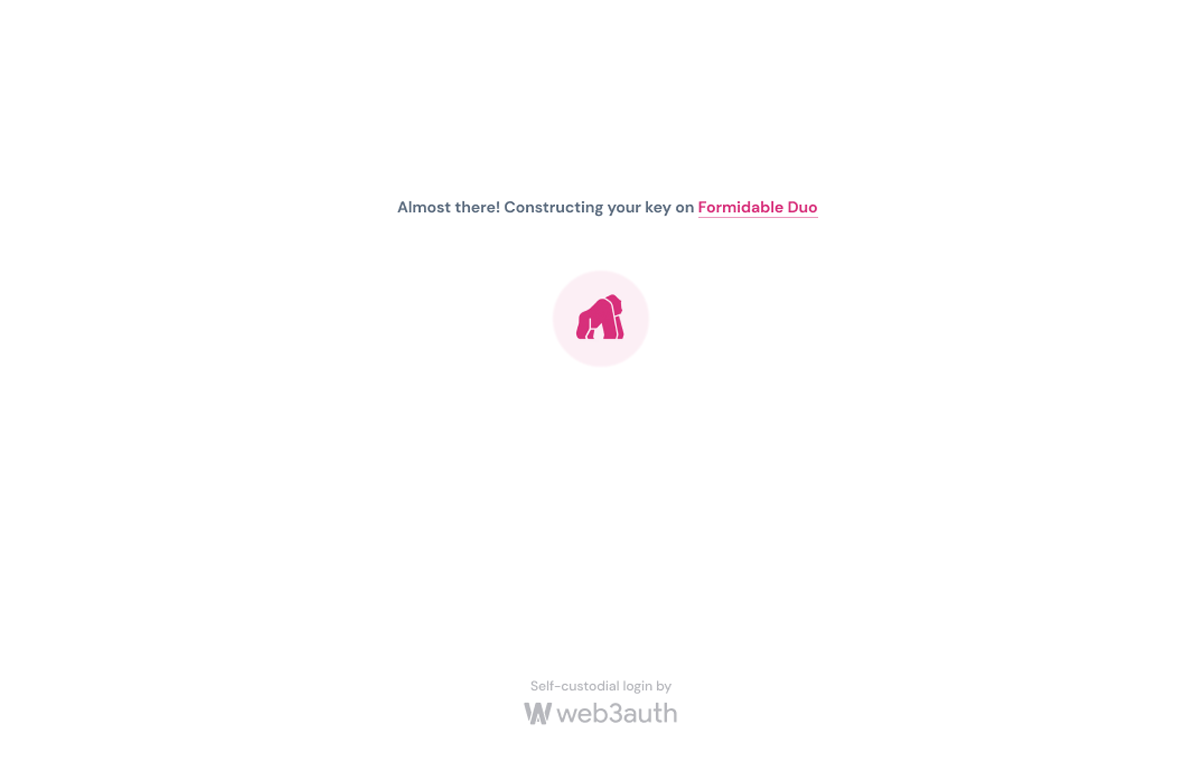
logoLight
& logoDark
The logo of the application. Displayed in dark and light mode respectively. This will be displayed in the key reconstruction page.
logoLight
on dark mode
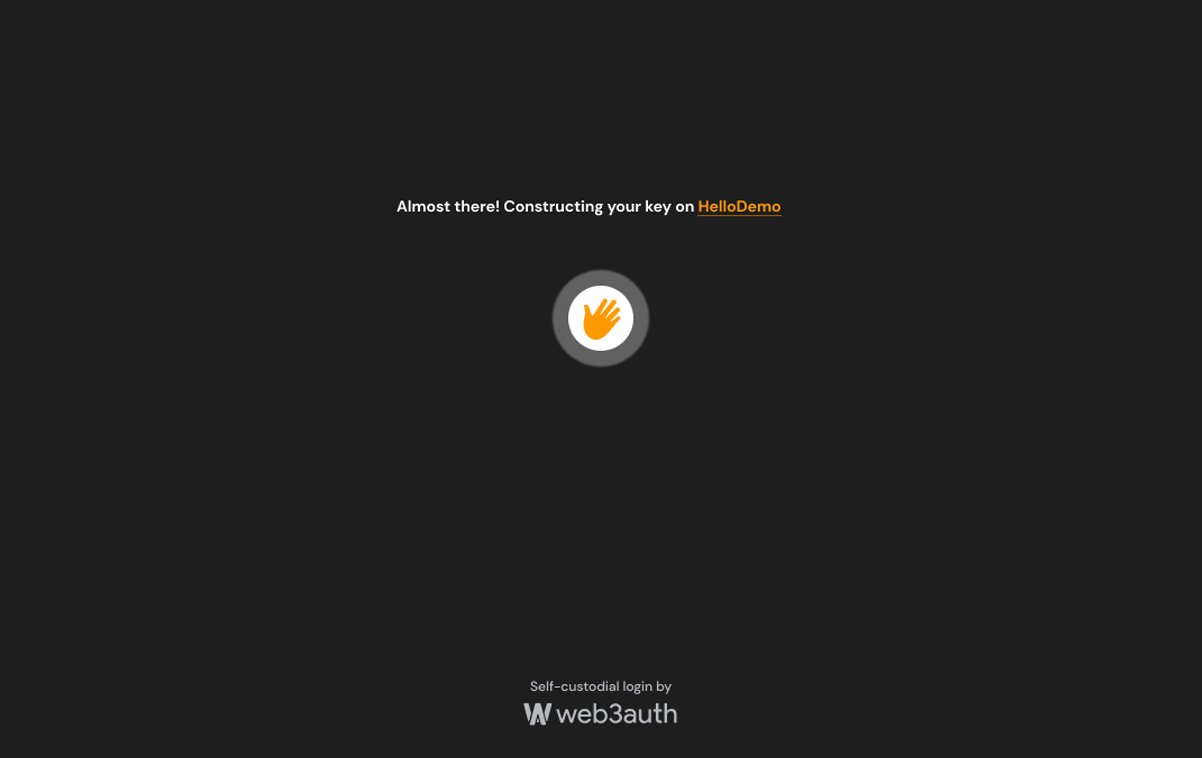
logoDark
on light mode
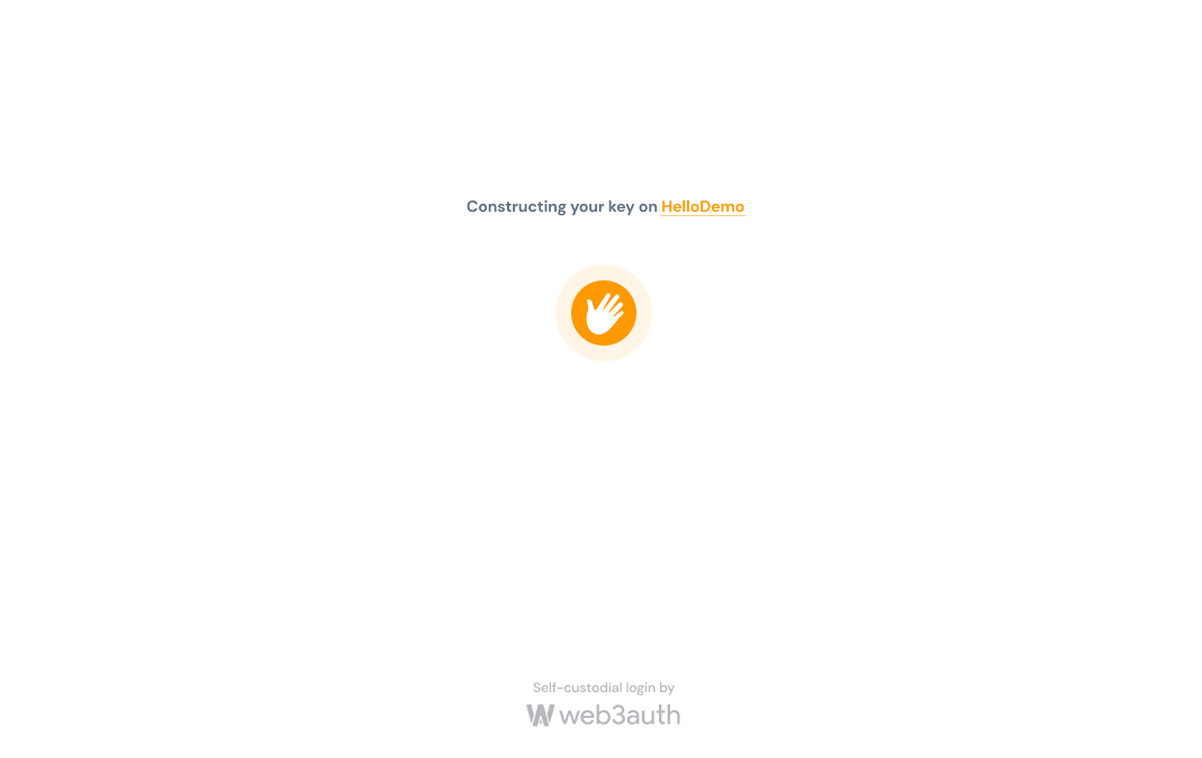
defaultLanguage
Default language will set the language used on all OpenLogin screens. The supported languages are:
en
- English (default)de
- Germanja
- Japaneseko
- Koreanzh
- Mandarines
- Spanishfr
- Frenchpt
- Portuguesenl
- Dutchtr
- Turkish
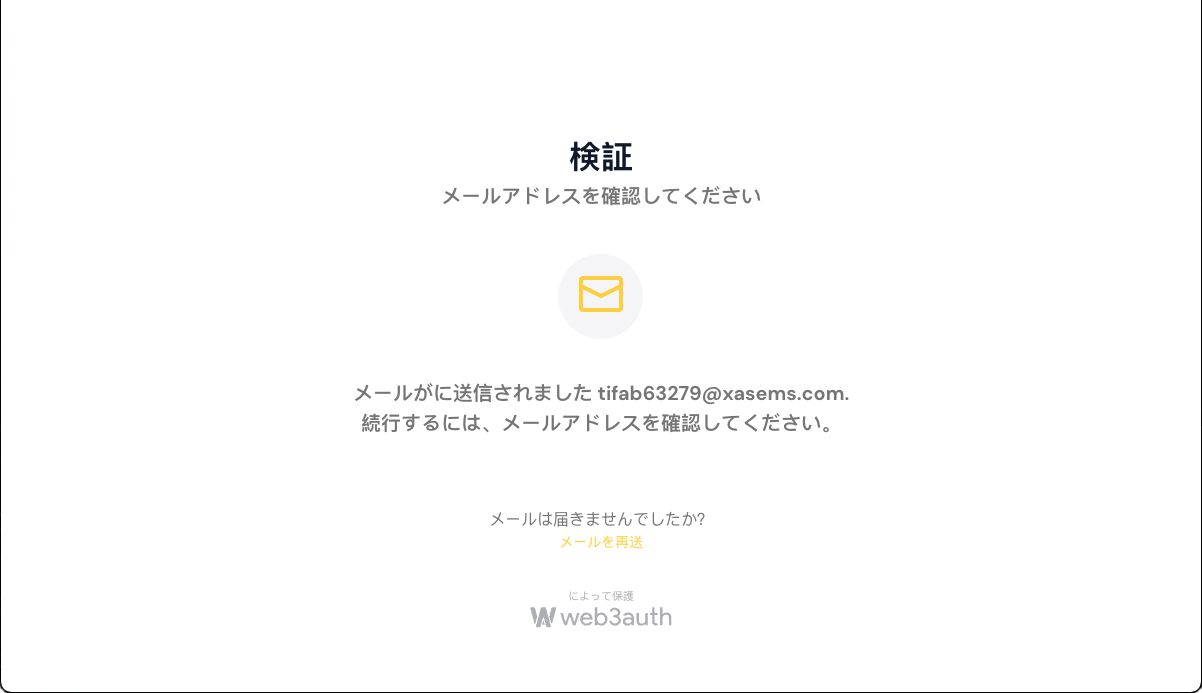
dark
Can be set to true
or false
with default set to false
.
For Light: dark: false
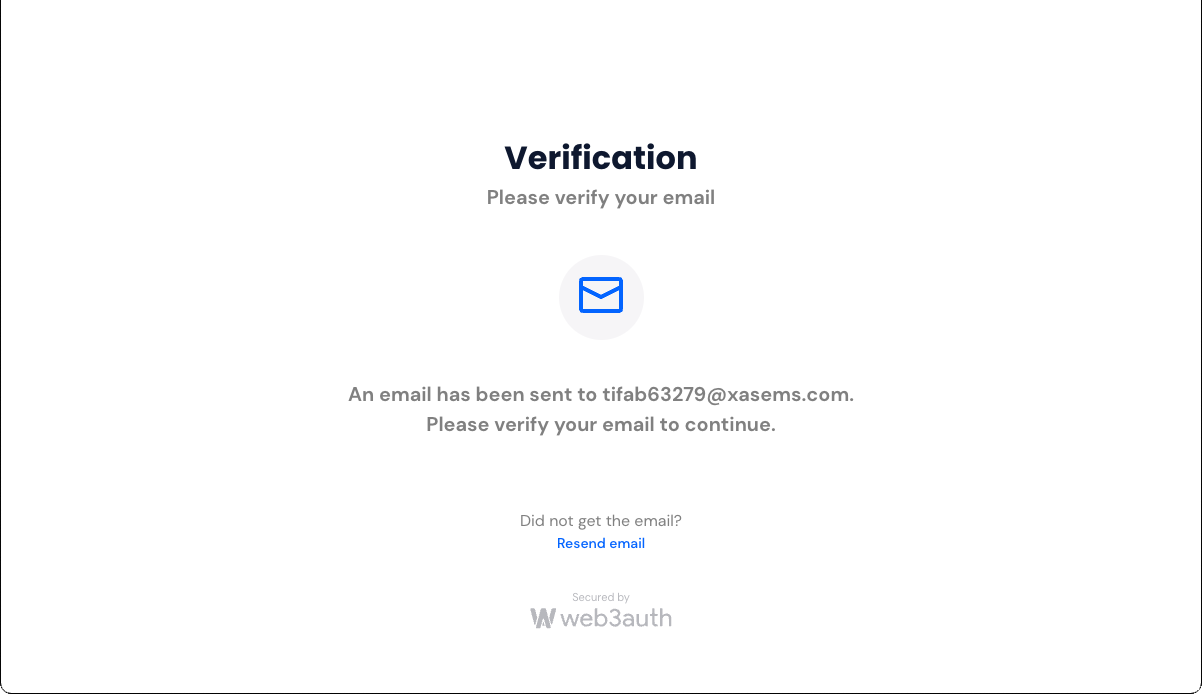
For Dark: dark: true
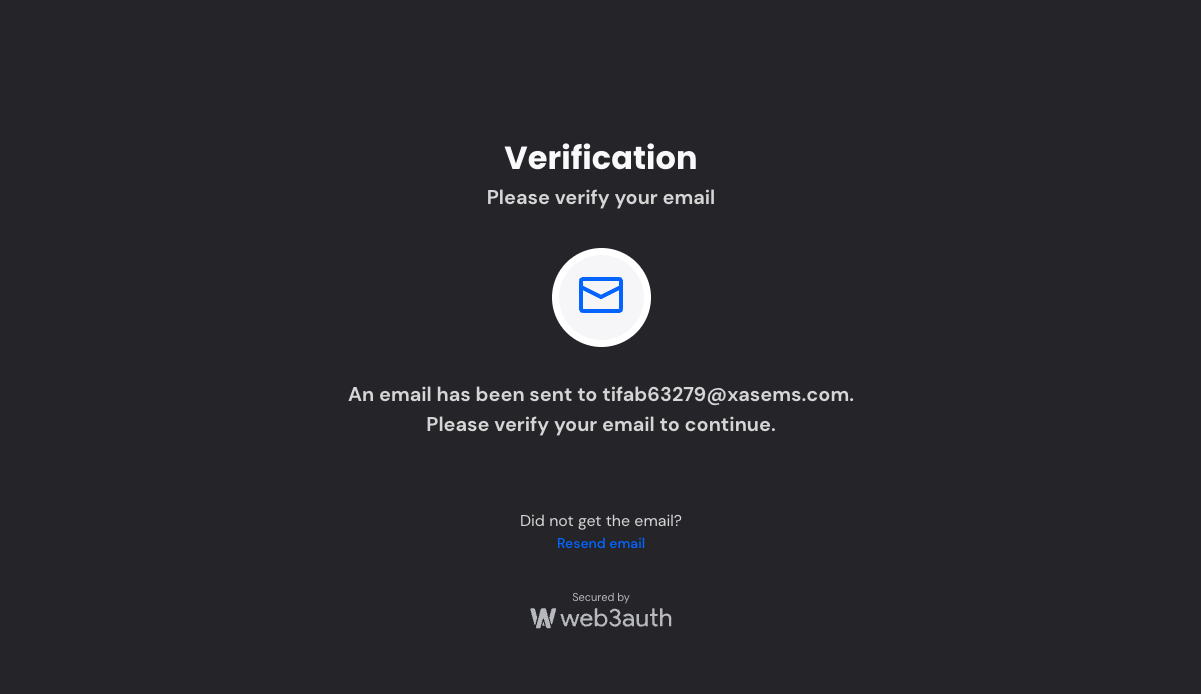
theme
Theme is a record of colors that can be configured. As of, now only primary
color can be set and
has effect on OpenLogin screens (default primary color is #0364FF
). Theme affects icons and links.
Examples below.
Standard color #0364FF
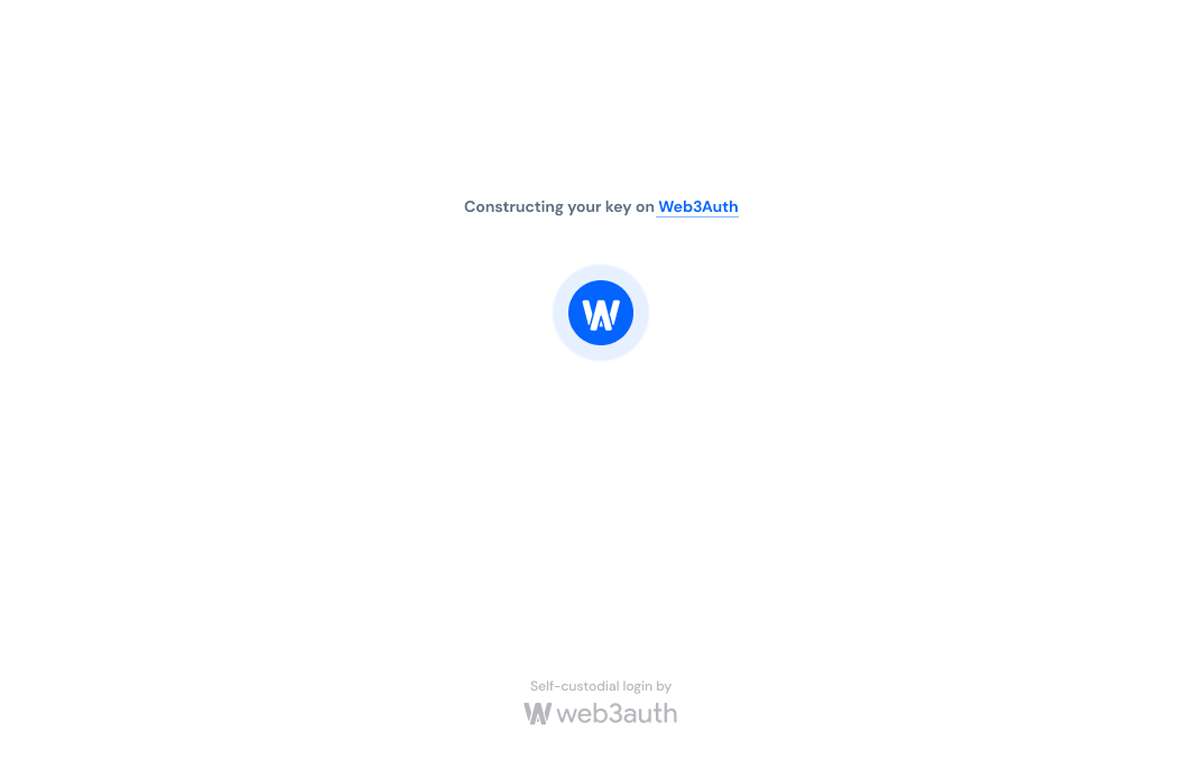
Color changed to #D72F7A
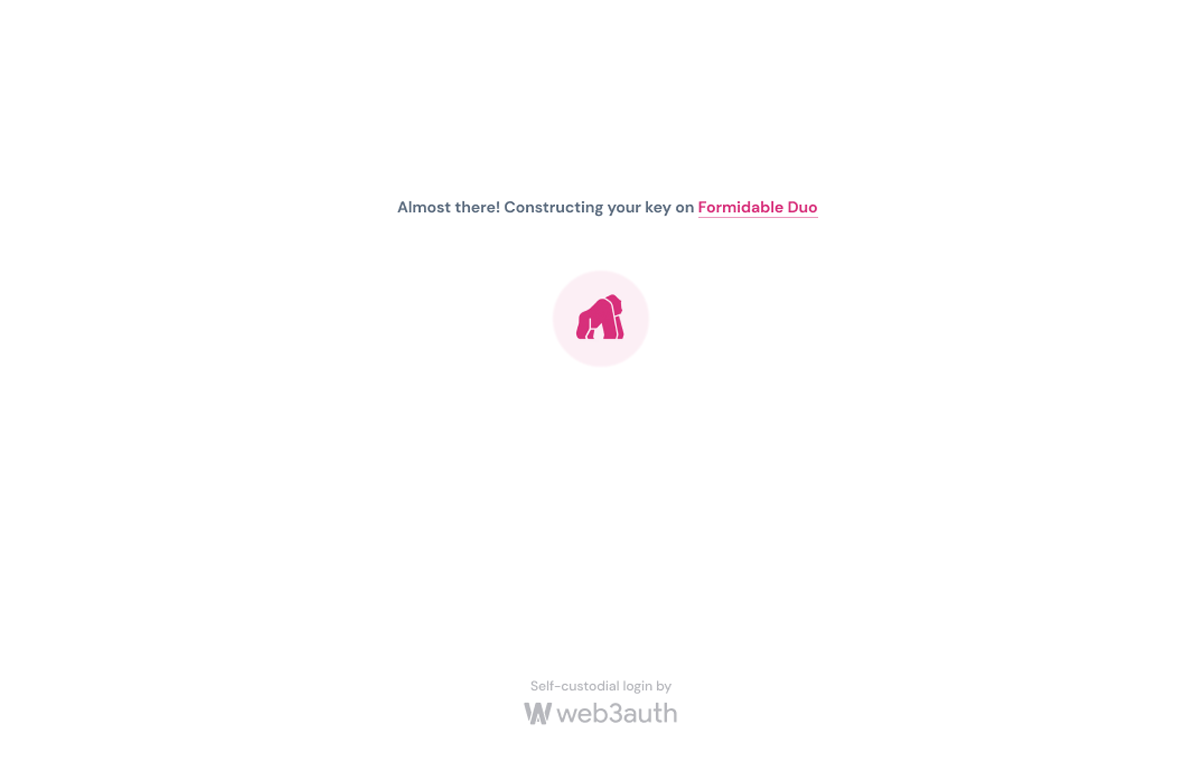
Example
- Expo Managed Workflow
- Bare React Native Workflow
import * as WebBrowser from "expo-web-browser";
import * as SecureStore from "expo-secure-store";
import Web3Auth, { LOGIN_PROVIDER, WEB3AUTH_NETWORK } from "@web3auth/react-native-sdk";
const clientId = "YOUR WEB3AUTH CLIENT ID";
const web3auth = new Web3Auth(WebBrowser, SecureStore, {
clientId,
network: WEB3AUTH_NETWORK.TESTNET, // or other networks
whiteLabel: {
appName: "My App",
logoLight: "https://web3auth.io/images/logo-light.png",
logoDark: "https://web3auth.io/images/logo-dark.png",
defaultLanguage: "en",
mode: "auto", // or "dark" or "light"
theme: {
primary: "#cddc39",
},
},
});
import * as WebBrowser from "@toruslabs/react-native-web-browser";
import EncryptedStorage from "react-native-encrypted-storage";
import Web3Auth, { LOGIN_PROVIDER, WEB3AUTH_NETWORK } from "@web3auth/react-native-sdk";
const clientId = "YOUR WEB3AUTH CLIENT ID";
const web3auth = new Web3Auth(WebBrowser, EncryptedStorage, {
clientId,
network: WEB3AUTH_NETWORK.TESTNET, // or other networks
whiteLabel: {
appName: "My App",
logoLight: "https://web3auth.io/images/logo-light.png",
logoDark: "https://web3auth.io/images/logo-dark.png",
defaultLanguage: "en",
mode: "auto", // or "dark" or "light"
theme: {
primary: "#cddc39",
},
},
});