Getting Started with Web3Auth Vue SDK
Overview
Web3Auth Plug and Play (PnP) provides a seamless authentication experience for Vue applications with social logins, external wallets, and more. Using our Vue Composables, you can easily connect users to their preferred wallets and manage authentication state.
Requirements
- This is a frontend SDK and must be used in a browser environment.
- Basic knowledge of JavaScript and Vue.
Installation
Install the Web3Auth Modal SDK using npm or yarn:
- npm
- Yarn
- pnpm
npm install --save @web3auth/modal
yarn add @web3auth/modal
pnpm add @web3auth/modal
Setup
Prerequisites Before you start, make sure you have registered on the Web3Auth Dashboard and have set up your project. You can look into the Dashboard Setup guide to learn more.
1. Configuration
Create a configuration file for Web3Auth. This file will contain your Web3Auth Client ID and Network details from the Web3Auth Dashboard.
import { type Web3AuthContextConfig } from "@web3auth/modal/vue";
import { WEB3AUTH_NETWORK, type Web3AuthOptions } from "@web3auth/modal";
const web3AuthOptions: Web3AuthOptions = {
clientId: "YOUR_CLIENT_ID", // Get your Client ID from Web3Auth Dashboard
web3AuthNetwork: WEB3AUTH_NETWORK.SAPPHIRE_MAINNET, // or WEB3AUTH_NETWORK.SAPPHIRE_DEVNET
};
const web3AuthContextConfig: Web3AuthContextConfig = {
web3AuthOptions,
};
export default web3AuthContextConfig;
2. Setup Web3Auth Provider
In your main component (e.g. App.vue
), import the Web3AuthProvider
component and wrap your
application with it:
<script setup lang="ts">
import Home from "./Home.vue";
import { Web3AuthProvider } from "@web3auth/modal/vue";
import web3AuthContextConfig from "./web3authContext";
</script>
<template>
<div class="min-h-screen flex flex-col">
<Web3AuthProvider :config="web3AuthContextConfig">
<Home />
</Web3AuthProvider>
</div>
</template>
Advanced Configuration
The Web3Auth Modal SDK offers a rich set of advanced configuration options:
- Smart Accounts: Configure account abstraction parameters.
- Custom Authentication: Define authentication methods.
- Whitelabeling & UI Customization: Personalize the modal's appearance.
- Multi-Factor Authentication (MFA): Set up and manage MFA.
- Wallet Services: Integrate additional wallet services.
Head over to the Advanced Configuration section to learn more about each configuration option.
Blockchain Integration
Web3Auth is blockchain agnostic, enabling integration with any blockchain network. Out of the box, Web3Auth offers robust support for both Solana and Ethereum, each with dedicated Vue composables.
Solana Integration
Solana composables are included natively within the @web3auth/modal
package. No additional setup
is required—simply use the provided composables to interact with Solana networks.
For detailed usage and examples, visit the Solana Composables section.
Ethereum Integration
Ethereum composables are provided through the popular wagmi
library, which works seamlessly with
Web3Auth. This allows you to leverage a wide range of Ethereum composables for account management,
transactions, and more.
For implementation details and examples, refer to the Ethereum Composables section.
- Basic Configuration
- Advanced Configuration
import { type Web3AuthContextConfig } from "@web3auth/modal/vue";
import { WEB3AUTH_NETWORK, type Web3AuthOptions } from "@web3auth/modal";
const web3AuthOptions: Web3AuthOptions = {
clientId: "YOUR_CLIENT_ID",
web3AuthNetwork: WEB3AUTH_NETWORK.SAPPHIRE_MAINNET, // or WEB3AUTH_NETWORK.SAPPHIRE_DEVNET
};
const web3AuthContextConfig: Web3AuthContextConfig = {
web3AuthOptions,
};
import { type Web3AuthContextConfig } from "@web3auth/modal/vue";
import {
WALLET_CONNECTORS,
WEB3AUTH_NETWORK,
MFA_LEVELS,
type Web3AuthOptions,
} from "@web3auth/modal";
const web3AuthOptions: Web3AuthOptions = {
clientId: "YOUR_CLIENT_ID",
web3AuthNetwork: WEB3AUTH_NETWORK.SAPPHIRE_MAINNET,
modalConfig: {
connectors: {
[WALLET_CONNECTORS.AUTH]: {
label: "auth",
loginMethods: {
google: {
name: "google login",
// logoDark: "url to your custom logo which will shown in dark mode",
},
facebook: {
name: "facebook login",
showOnModal: false, // hides the facebook option
},
email_passwordless: {
name: "email passwordless login",
showOnModal: true,
authConnectionId: "w3a-email_passwordless-demo",
},
sms_passwordless: {
name: "sms passwordless login",
showOnModal: true,
authConnectionId: "w3a-sms_passwordless-demo",
},
},
showOnModal: true, // set to false to hide all social login methods
},
},
hideWalletDiscovery: true, // set to true to hide external wallets discovery
},
mfaLevel: MFA_LEVELS.MANDATORY,
};
const web3AuthContextConfig: Web3AuthContextConfig = {
web3AuthOptions,
};
Troubleshooting
Bundler Issues: Missing Dependencies
You might encounter errors related to missing dependencies in the browser environment:
Buffer is not defined
process is not defined
- Other Node.js-specific modules missing errors
These Node.js dependencies need to be polyfilled in your application. We've prepared detailed troubleshooting guides for popular bundlers:
- Vite Troubleshooting Guide
- Svelte Troubleshooting Guide
- Nuxt Troubleshooting Guide
- Webpack 5 Troubleshooting Guide
JWT Errors
When using Custom Authentication, you may encounter JWT errors:
- Invalid JWT Verifiers ID field
- Failed to verify JWS signature
- Duplicate Token
- Expired Token
- Mismatch JWT Validation field
Different Private Keys Across Integrations
If you're getting different private keys across integrations, see our guide on Different Private Keys across integrations.
Quick Starts
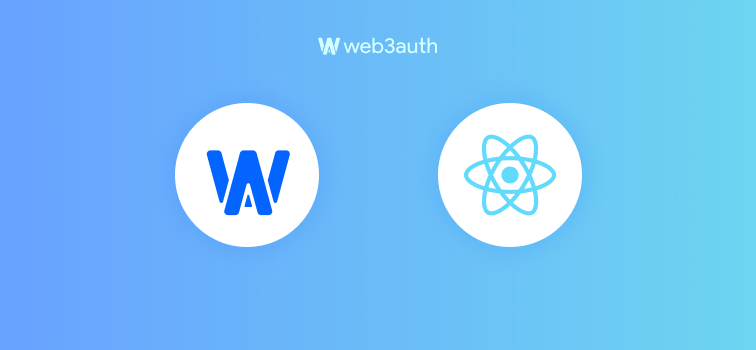
React Quick Start
A quick integration of Web3Auth SDK in React
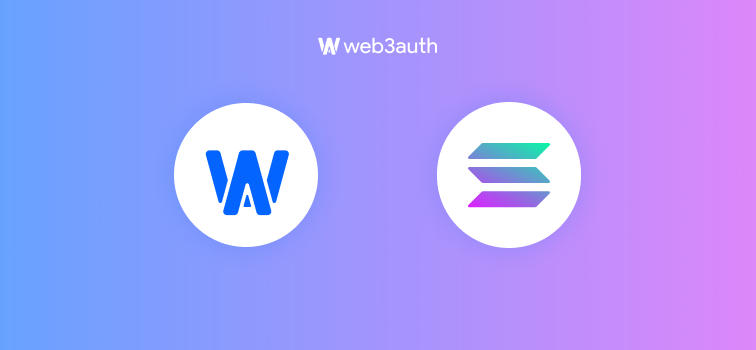
React Solana Quick Start
A quick integration of Web3Auth SDK in React with Solana blockchain
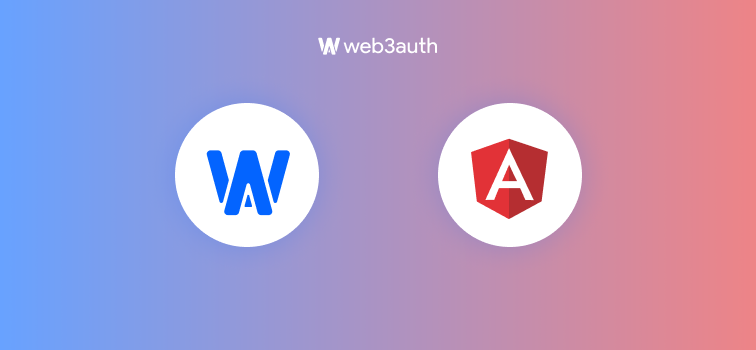
Angular Quick Start
A quick integration of Web3Auth SDK in Angular
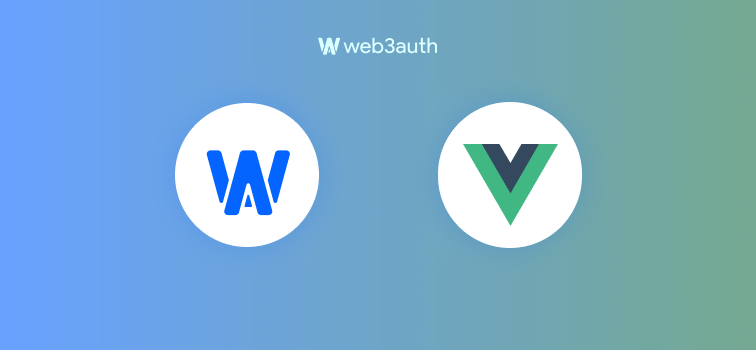
Vue Quick Start
A quick integration of Web3Auth SDK in Vue
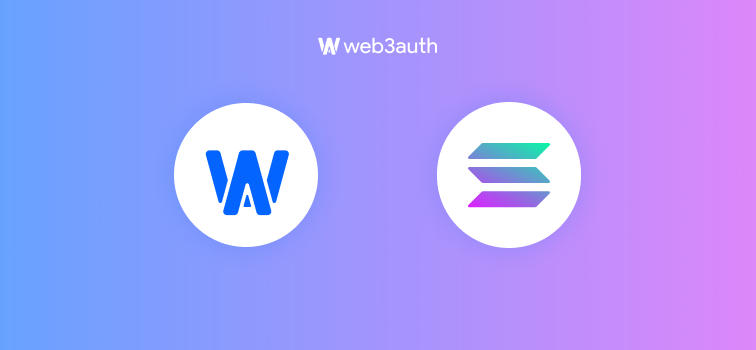
Vue Solana Quick Start
A quick integration of Web3Auth SDK in Vue with Solana blockchain
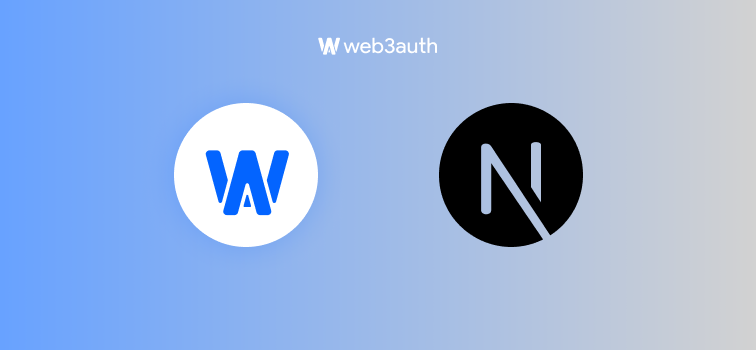
NextJS Quick Start
A quick integration of Web3Auth SDK in NextJS