Getting Started with Web3Auth SDK
Overview
Web3Auth Plug and Play (PnP) provides a seamless authentication experience for web applications with social logins, external wallets, and more. Using our JavaScript SDK, you can easily connect users to their preferred wallets and manage authentication state.
Requirements
- This is a frontend SDK and must be used in a browser environment.
- Basic knowledge of JavaScript.
Installation
Install the Web3Auth Modal SDK using npm or yarn:
- npm
- Yarn
- pnpm
- Bun
npm install --save @web3auth/modal
yarn add @web3auth/modal
pnpm add @web3auth/modal
bun add @web3auth/modal
Setup
Prerequisites Before you start, make sure you have registered on the Web3Auth Dashboard and have set up your project. You can look into the Dashboard Setup guide to learn more.
1. Configuration
Create an instance of Web3Auth containing the basic needed configuration. These configuration will contain your Web3Auth Client ID and Network details from the Web3Auth Dashboard.
import { Web3Auth, WEB3AUTH_NETWORK } from "@web3auth/modal";
const web3auth = new Web3Auth({
clientId: "YOUR_CLIENT_ID", // Get your Client ID from Web3Auth Dashboard
web3AuthNetwork: WEB3AUTH_NETWORK.SAPPHIRE_MAINNET, // or WEB3AUTH_NETWORK.SAPPHIRE_DEVNET
});
2. Initialize Web3Auth
Initialize the Web3Auth instance before using any authentication methods:
await web3auth.init();
Advanced Configuration
The Web3Auth Modal SDK offers a rich set of advanced configuration options:
- Smart Accounts: Configure account abstraction parameters.
- Custom Authentication: Define authentication methods.
- Whitelabeling & UI Customization: Personalize the modal's appearance.
- Multi-Factor Authentication (MFA): Set up and manage MFA.
- Wallet Services: Integrate additional wallet services.
Head over to the Advanced Configuration section to learn more about each configuration option.
Blockchain Integration
Web3Auth is blockchain agnostic, enabling integration with any blockchain network. Out of the box, Web3Auth offers robust support for both Solana and Ethereum, each with dedicated provider methods.
Solana Integration
To interact with Solana networks, you can get the provider from Web3Auth and use it with Solana libraries:
await web3auth.connect();
// Use with a Solana library
const solanaWallet = new SolanaWallet(web3auth.provider);
Ethereum Integration
For Ethereum integration, you can get the provider and use it with ethers or viem:
await web3auth.connect();
// Use with ethers.js
const ethProvider = new ethers.BrowserProvider(web3auth.provider);
// OR
// Use with viem
const walletClient = createWalletClient({
chain: getViewChain(web3auth.provider),
transport: custom(web3auth.provider),
});
- Basic Configuration
- Advanced Configuration
import { Web3Auth, WEB3AUTH_NETWORK } from "@web3auth/modal";
const web3auth = new Web3Auth({
clientId: "YOUR_CLIENT_ID",
web3AuthNetwork: WEB3AUTH_NETWORK.SAPPHIRE_MAINNET, // or WEB3AUTH_NETWORK.SAPPHIRE_DEVNET
});
import { Web3Auth, WEB3AUTH_NETWORK, WALLET_CONNECTORS, MFA_LEVELS } from "@web3auth/modal";
const web3auth = new Web3Auth({
clientId: "YOUR_CLIENT_ID",
web3AuthNetwork: WEB3AUTH_NETWORK.SAPPHIRE_MAINNET,
modalConfig: {
connectors: {
[WALLET_CONNECTORS.AUTH]: {
label: "auth",
loginMethods: {
google: {
name: "google login",
// logoDark: "url to your custom logo which will shown in dark mode",
},
facebook: {
name: "facebook login",
showOnModal: false, // hides the facebook option
},
email_passwordless: {
name: "email passwordless login",
showOnModal: true,
authConnectionId: "w3a-email_passwordless-demo",
},
sms_passwordless: {
name: "sms passwordless login",
showOnModal: true,
authConnectionId: "w3a-sms_passwordless-demo",
},
},
showOnModal: true, // set to false to hide all social login methods
},
},
hideWalletDiscovery: true, // set to true to hide external wallets discovery
},
mfaLevel: MFA_LEVELS.MANDATORY,
});
Troubleshooting
Bundler Issues: Missing Dependencies
You might encounter errors related to missing dependencies in the browser environment:
Buffer is not defined
process is not defined
- Other Node.js-specific modules missing errors
These Node.js dependencies need to be polyfilled in your application. We've prepared detailed troubleshooting guides for popular bundlers:
- Vite Troubleshooting Guide
- Svelte Troubleshooting Guide
- Nuxt Troubleshooting Guide
- Webpack 5 Troubleshooting Guide
JWT Errors
When using Custom Authentication, you may encounter JWT errors:
- Invalid JWT Verifiers ID field
- Failed to verify JWS signature
- Duplicate Token
- Expired Token
- Mismatch JWT Validation field
Different Private Keys Across Integrations
If you're getting different private keys across integrations, see our guide on Different Private Keys across integrations.
Quick Starts
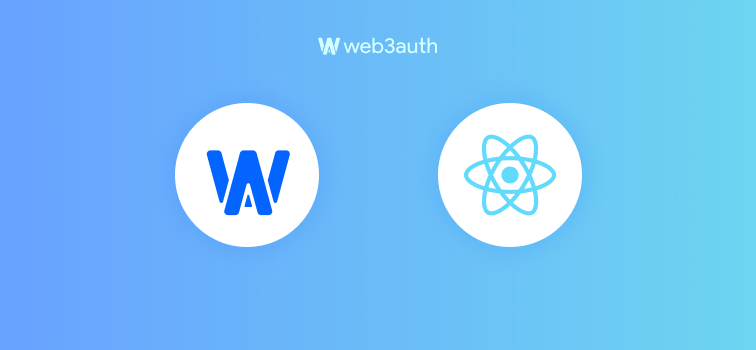
React Quick Start
A quick integration of Web3Auth SDK in React
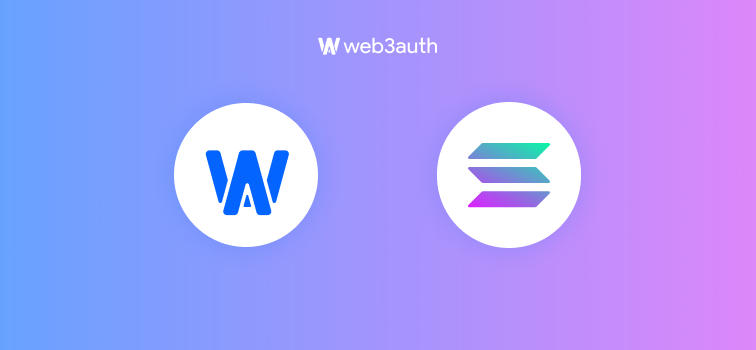
React Solana Quick Start
A quick integration of Web3Auth SDK in React with Solana blockchain
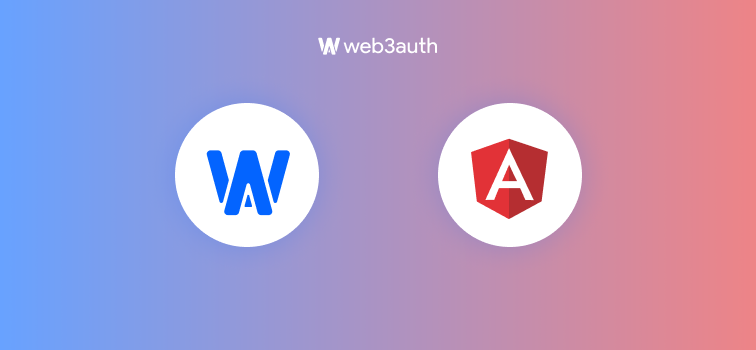
Angular Quick Start
A quick integration of Web3Auth SDK in Angular
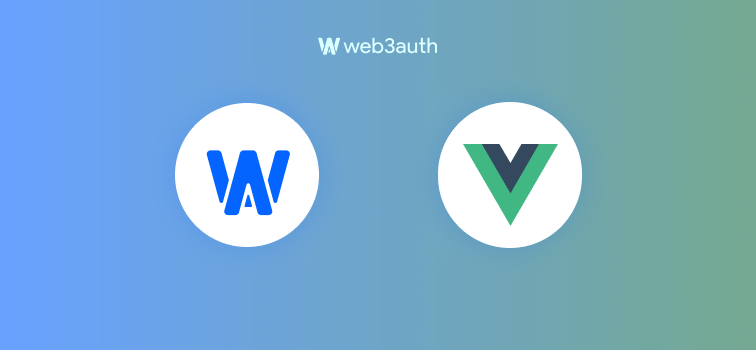
Vue Quick Start
A quick integration of Web3Auth SDK in Vue
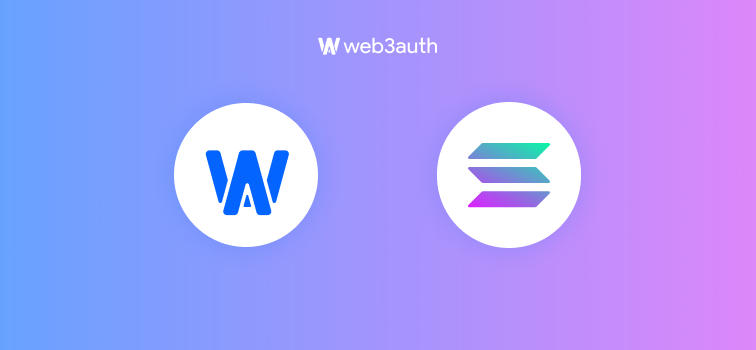
Vue Solana Quick Start
A quick integration of Web3Auth SDK in Vue with Solana blockchain
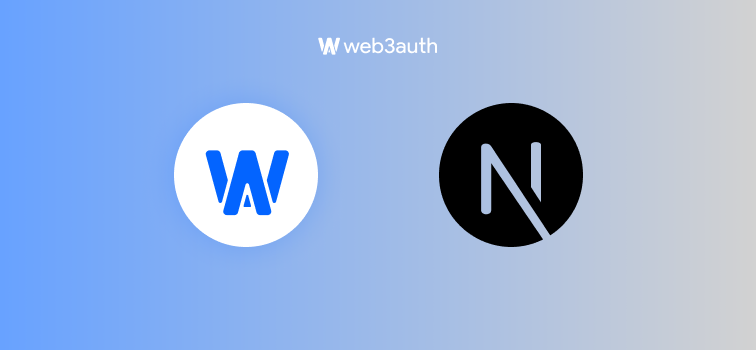
NextJS Quick Start
A quick integration of Web3Auth SDK in NextJS