How to integrate Web3Auth Unity SDK
This guide will cover the basics of how to use the Web3Auth Unity SDK in your preferred platform. Before starting you can try out the sample application as mentioned in quick start.
Quick Start
npx degit Web3Auth/web3auth-pnp-examples/unity/unity-quick-start w3a-unity-demo
# Open in Unity Hub
How it works?
When integrating Web3Auth Unity SDK with Social Login the flow looks something like this:
-
When a user logs in with a login provider like
Google
, it sends a JWTid_token
to the app. This JWT token is sent to the Web3Auth SDK's login function. -
Finally, on successful validation of the JWT token, Web3Auth SDK will generate a private key for the user, in a self custodial way, resulting in easy onboarding for your user to the application.
Prerequisites
-
For Web Apps: A basic knowledge of JavaScript is required to use Web3Auth SDK.
-
For Mobile Apps: For the Web3Auth Mobile SDKs, you have a choice between iOS, Android, React Native & Flutter. Please refer to the Web3Auth SDK Reference for more information.
-
Create a Web3Auth account on the Web3Auth Dashboard
-
Unity Editor 2019.4.9f1 or greater
-
.Net Framework 4.x
Setup
Setup your Web3Auth Dashboard
-
Create a Project from the Project Section of the Web3Auth Developer Dashboard.
-
Enter your desired Project name.
-
Select the Product you want to use. For this guide, we'll be using the Plug n Play product.
-
Select the Platform type you want to use. For this guide, we'll be using the Web Application as the platform.
-
Select the Web3Auth Network as
Sapphire Devnet
. We recommend creating a project in thesapphire_devnet
network during development. While moving to a production environment, make sure to convert your project tosapphire_mainnet
or any of the legacy mainnet networkmainnet
,aqua
, orcyan
network. Otherwise, you'll end up losing users and keys. -
Select the blockchain(s) you'll be building this project on. For interoperability with Torus Wallets, you have the option of allowing the user's private key to be used in other applications using Torus Wallets (EVM, Solana, XRPL & Casper).
-
Finally, once you create the project, you have the option to whitelist your URLs for the project. Please whitelist the domains where your project will be hosted.
-
-
Add
{{SCHEMA}}://{YOUR_APP_PACKAGE_NAME}://auth
to Whitelist URLs field of the Web3Auth Dashboard. -
Copy the
Client ID
for usage later.
Using the Web3Auth SDK
To use the Web3Auth SDK, you need to add the dependency of the respective platform SDK of Web3Auth to your project. To know more about the available SDKs, please have a look at this documentation page.
For this guide here, we will be talking through the Web3Auth Unity SDK.
Installation
Download .unitypackage from our latest release and import the package file into your existing Unity3D project.
You may encounter errors when importing this package into your existing project.
The type or namespace name 'Newtonsoft' could not be found (are you missing a using directive or an assembly reference?)
To fix this problem you need to add the following line into dependencies object which is inside the
Packages/manifest.json
file.
"com.unity.nuget.newtonsoft-json": "3.2.1"
Configure Deep Link (for Android and iOS)
Unity SDK works on unity deep linking features to redirect the callback from Web3Auth. Before building the application for Android/IOS you need to register the redirect_uri that can be done easily by the tool provided inside the SDK. To achieve that, you need to follow the steps mentioned below.
-
Open deep link generator tool provided by Web3Auth Unity SDK from
Window > Web3Auth > Deep Link Generator
-
Enter the
redirect_url
{{SCHEMA}}://{YOUR_APP_PACKAGE_NAME}://auth
and click generate.
We're using torusapp://com.torus.Web3AuthUnity/auth
as the redirect_url
in our example just to
give you a reference on how your app's redirect_url
should look like.
Initialization
After Installation, the next step to using Web3Auth is to Initialize the SDK.
However, Initialization is a two-step process:
Please note that these are the most critical steps where you must pass on different parameters according to the preference of your project. Additionally, You must configure Whitelabeling and Custom Authentication within this step if you wish to customize your Web3Auth Instance.
Create Web3Auth Instance
Attach a Web3Auth.cs
script to your game object where you are planning to write your
authentication code.
You can refer to following sample file on how your boilerplate script should look like:
using System;
using System.Linq;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.UI;
using Newtonsoft.Json;
public class Web3Auth : MonoBehaviour
{
// Start is called before the first frame update
void Start() {}
public void login() {}
private void onLogin(Web3AuthResponse response) {}
public void logout() {}
private void onLogout() {}
}
Within your script, import the Web3Auth
component in your class.
Web3Auth web3Auth;
Next create an instance within your Start()
function by creating an instance of the component you
just imported.
web3Auth = GetComponent<Web3Auth>();
Setting up Web3Auth Options
After instantiation, within your Start()
function, set up the Web3Auth Options as follows:
web3Auth.setOptions(new Web3AuthOptions()
{
redirectUrl = new Uri("torusapp://com.torus.Web3AuthUnity/auth"),
clientId = "BAwFgL-r7wzQKmtcdiz2uHJKNZdK7gzEf2q-m55xfzSZOw8jLOyIi4AVvvzaEQO5nv2dFLEmf9LBkF8kaq3aErg",
network = Web3Auth.Network.TESTNET,
});
You can also configure your client_id
, redirect_url
and network
within the script setting in
Unity Editor. It will look something like this:
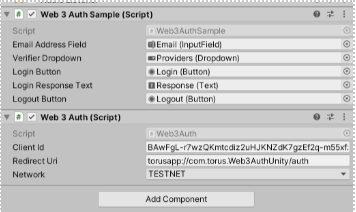
Authentication
Once you've installed and successfully initialized Web3Auth, you can use it to authenticate your users.
Logging in
The web3Auth.login(LoginParams)
function helps your user to trigger the login process. The login
flow will be triggered based on the selected provider.
public void login()
{
var selectedProvider = Provider.GOOGLE;
var options = new LoginParams()
{
loginProvider = selectedProvider
};
web3Auth.login(options);
}
When connecting, the login
function takes the LoginParams arguments for the login. See the
LoginParams for more details.