Auth0 Login with Web3Auth
Auth0 is a powerful authentication and authorization platform that enables developers to securely manage user identities. Web3Auth offers native support for integrating Auth0 as a service provider, allowing projects to leverage Auth0’s robust authentication mechanisms within the Web3Auth ecosystem.
Take a look at the supported social logins on Auth0
Create an Auth0 Application
To begin, developers must first create an Auth0 application specific to their project. This initial setup is essential before configuring the connection with Web3Auth. Once the Auth0 application is created, developers can proceed to establish an Auth0 connection within the Web3Auth Dashboard.
This integration allows users to authenticate through Auth0, while still benefiting from Web3Auth’s key management and wallet abstraction features. For platform-specific implementation details or additional customization, developers are encouraged to refer to the official Auth0 documentation.
Create an Auth0 Connection
To use this feature, developers must go to the Custom Connections
tab in the
Web3Auth Dashboard.
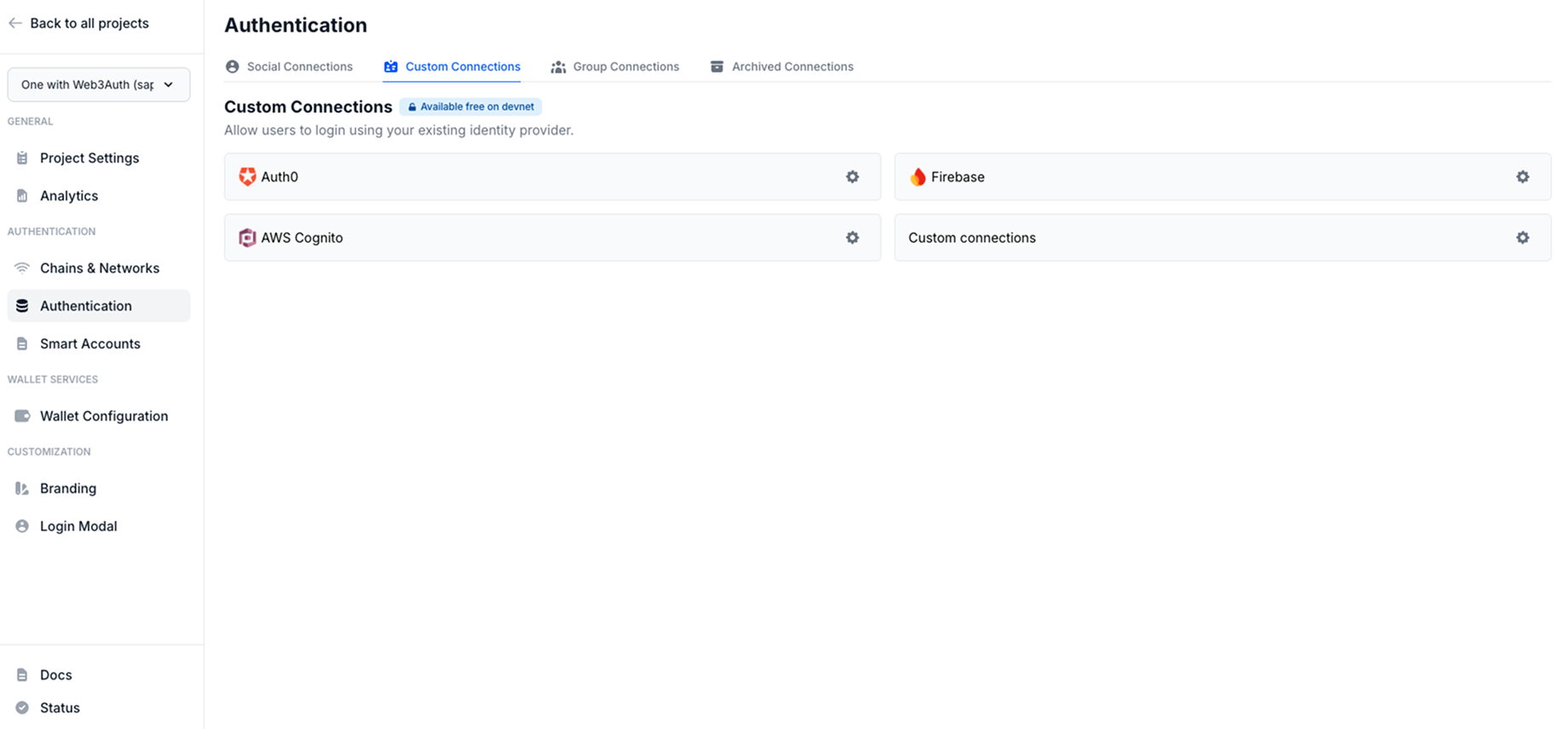
Follow these steps to create an Auth0 connection:
- Visit the Web3Auth Dashboard.
- Go to the
Custom Connections
section. - Click on the
Settings
icon near the Auth0 connection. - Enter the
Auth Connection ID
. - Select the
JWT user identifier
:email
,sub
orcustom
. - Toggle the Case Sensitivity of
User Identifier
. (Optional) - Enter
Auth0 Client ID
. - Enter
Auth0 Domain
. - Finally, click on the
Add Connection
button.
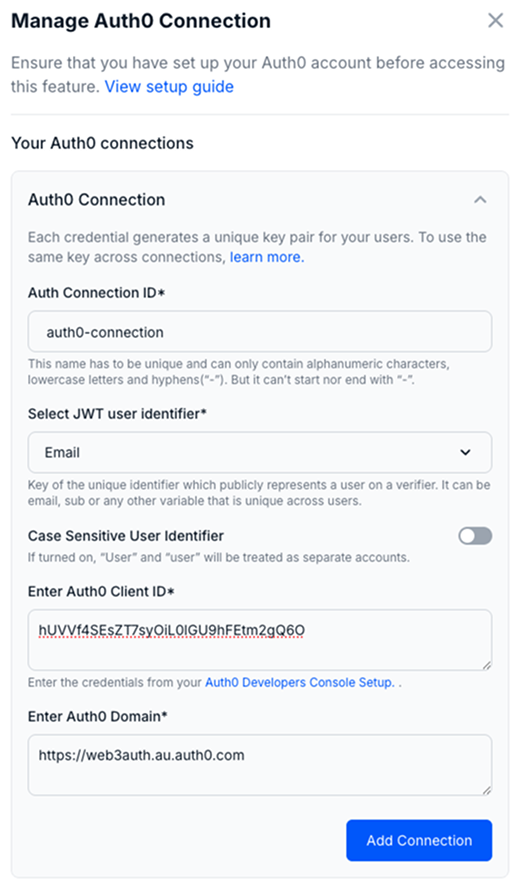
Usage
Since, the Auth0 Connection
details are available from Dashboard, developers don't need to pass
any additional parameters to the Web3AuthProvider
.
Follow our Quickstart Guide to setup the basic flow.
Web
- Implicit Login (SPA)
- Implicit Login Auth0 Google
- JWT Login with Auth0 SDK
await connectTo(WALLET_CONNECTORS.AUTH, {
authConnection: AUTH_CONNECTION.CUSTOM,
authConnectionId: "w3a-auth0-demo",
});
await connectTo(WALLET_CONNECTORS.AUTH, {
authConnection: AUTH_CONNECTION.CUSTOM,
authConnectionId: "w3a-auth0-demo",
extraLoginOptions: {
connection: "google-oauth2",
},
});
const { getIdTokenClaims, loginWithPopup } = useAuth0();
const loginWithAuth0 = async () => {
try {
await loginWithPopup();
const idToken = (await getIdTokenClaims())?.__raw.toString();
if (!idToken) {
throw new Error("No id token found");
}
await connectTo(WALLET_CONNECTORS.AUTH, {
authConnectionId: "w3a-auth0-demo",
authConnection: AUTH_CONNECTION.CUSTOM,
idToken,
extraLoginOptions: {
isUserIdCaseSensitive: false,
},
});
} catch (err) {
console.error(err);
}
};
Android
Create Web3Auth Instance
In your activity, create a Web3Auth
instance with your Web3Auth project's configurations.
web3Auth = Web3Auth(
Web3AuthOptions(
context = this,
clientId = getString(R.string.web3auth_project_id), // pass over your Web3Auth Client ID from Developer Dashboard
network = Network.SAPPHIRE_MAINNET
redirectUrl = Uri.parse("{YOUR_APP_PACKAGE_NAME}://auth"), // your app's redirect URL
loginConfig = hashMapOf("jwt" to LoginConfigItem(
verifier = "web3auth-auth0-demo",
typeOfLogin = TypeOfLogin.JWT,
name = "Auth0 Login",
clientId = getString(R.string.web3auth_auth0_client_id)
)
)
)
)
// Handle user signing in when app is not alive
web3Auth.setResultUrl(intent?.data)
Logging in
Once initialized, you can use the web3Auth.login(LoginParams("{selectedLoginProvider}"))
function
to authenticate the user when they click the login button.
private fun signIn() {
val selectedLoginProvider = Provider.JWT // For Auth0, we use JWT
val loginCompletableFuture: CompletableFuture<Web3AuthResponse> = web3Auth.login(
LoginParams(
selectedLoginProvider,
extraLoginOptions = ExtraLoginOptions(
domain = "https://YOUR_AUTH0_DOMAIN",
verifierIdField = "sub"
)
)
)
}
When connecting, the login
function takes the LoginParams arguments for the login. See the
LoginParams for more details.
Flutter
Create Web3Auth Instance
In your main.dart
file, initialize the Web3AuthFlutter
plugin at the very beginning such as in
the overriden initState
function
Future<void> initPlatformState() async {
Uri redirectUrl;
if (Platform.isAndroid) {
redirectUrl = Uri.parse('{SCHEME}://{HOST}/auth');
// w3a://com.example.w3aflutter/auth
} else if (Platform.isIOS) {
redirectUrl = Uri.parse('{bundleId}://auth');
// com.example.w3aflutter://openlogin
} else {
throw UnKnownException('Unknown platform');
}
final loginConfig = HashMap<String, LoginConfigItem>();
loginConfig['jwt'] = LoginConfigItem(
verifier: "VERIFIER-NAME", // get it from web3auth dashboard
typeOfLogin: TypeOfLogin.jwt,
name: "Web3Auth Flutter Auth0 Example",
clientId: "AUTH0-CLIENT-ID" // auth0 client id
);
await Web3AuthFlutter.init(Web3AuthOptions(
clientId:'YOUR WEB3AUTH CLIENT ID FROM DASHBOARD',
network: Network.sapphire_mainnet,
redirectUri: redirectUrl,
loginConfig: loginConfig
));
await Web3AuthFlutter.initialize();
}
Logging in
Once initialized, you can use the
Web3AuthFlutter.login(LoginParams( loginProvider: Provider.google ))
function to authenticate the
user when they click the login button.
Future<Web3AuthResponse> _withAuth0() {
return Web3AuthFlutter.login(LoginParams(
loginProvider: Provider.jwt,
mfaLevel: MFALevel.OPTIONAL,
extraLoginOptions: ExtraLoginOptions(
domain: 'YOUR_AUTH0_DOMAIN', // eg. https://torus.us.auth0.com
verifierIdField: 'sub')));
}
Read more about initializing the Flutter SDK here.
React Native
const web3auth = new Web3Auth(WebBrowser, SecureStore, {
clientId,
network: OPENLOGIN_NETWORK.SAPPHIRE_MAINNET, // SAPPHIRE_MAINNET or SAPPHIRE_DEVNET
loginConfig: {
jwt: {
verifier: "YOUR_AUTH0_VERIFIER_NAME", // Please create a verifier on the developer dashboard and pass the name here
typeOfLogin: "jwt",
clientId: "AUTH0_CLIENT_ID_123ABcdefg4HiJKlMno4P5QR6stUvWXY", // use your app client id you got from auth0
},
},
});
const web3authResponse = await web3auth.login({
redirectUrl: resolvedRedirectUrl,
loginProvider: "jwt",
extraLoginOptions: {
domain: "https://YOUR-AUTH0-DOMAIN", // Please append "https://" before your domain
verifierIdField: "sub", // For SMS & Email Passwordless, use "name" as verifierIdField
},
});