Initializing PnP Web No Modal SDK
After Installation, the next step to use Web3Auth is to Initialize the SDK.
- Instantiation of Web3AuthNoModal
- Configuration of Adapters
- Configuration of Plugins (optional)
- Initialization of Web3Auth
Please note that these are the most critical steps where you need to pass on different parameters according to the preference of your project. Additionally, Whitelabeling, Multi Factor Authentication and Custom Authentication have to be configured within this step, if you wish to customize your Web3Auth Instance.
Instantiating Web3AuthNoModal
Import the Web3AuthNoModal
class from @web3auth/no-modal
import { Web3AuthNoModal } from "@web3auth/no-modal";
Assign the Web3AuthNoModal
class to a variable
const web3auth = new Web3AuthNoModal(IWeb3AuthCoreOptions);
This Web3AuthNoModal constructor takes an object with IWeb3AuthCoreOptions
as input.
Arguments
IWeb3AuthCoreOptions
- Table
- Interface
Parameter | Description |
---|---|
clientId | Client id for web3auth. You can obtain your client id from the web3auth developer dashboard. You can set any random string for this on localhost. |
chainConfig | Custom chain configuration for chainNamespace. |
enableLogging | Setting to true will enable logs. |
storageKey | Setting to "local" will persist social login session across browser tabs. |
sessionTime | SessionTime (in seconds) for idToken issued by Web3Auth for server-side verification. |
web3AuthNetwork | Web3Auth Network to use for the session & the issued idToken. |
useCoreKitKey | Uses core-kit key with Web3Auth provider. |
uiConfig | WhiteLabel options for Web3Auth. |
privateKeyProvider | Private key provider for your chain namespace. |
export interface IWeb3AuthCoreOptions {
/**
* Client id for web3auth.
* You can obtain your client id from the web3auth developer dashboard.
* You can set any random string for this on localhost.
*/
clientId: string;
/**
* Custom chain configuration for chainNamespace.
*
* @defaultValue mainnet config of provided chainNamespace.
*/
chainConfig?: CustomChainConfig;
/**
* Setting to true will enable logs.
*
* @defaultValue false.
*/
enableLogging?: boolean;
/**
* Setting to "local" will persist social login session across browser tabs.
*
* @defaultValue "local".
*/
storageKey?: "session" | "local";
/**
* SessionTime (in seconds) for idToken issued by Web3Auth for server-side verification.
* @defaultValue 86400.
*
* Note: max value can be 30 days (86400 * 30) and min can be 1 day (86400).
*/
sessionTime?: number;
/**
* Web3Auth Network to use for the session & the issued idToken.
* @defaultValue mainnet.
*/
web3AuthNetwork?: WEB3AUTH_NETWORK_TYPE;
/**
* Uses core-kit key with Web3Auth provider.
* @defaultValue false.
*/
useCoreKitKey?: boolean;
/**
* WhiteLabel options for Web3Auth.
*/
uiConfig?: WhiteLabelData;
/**
* Private key provider for your chain namespace.
*/
privateKeyProvider?: IBaseProvider<string>;
}
export type WEB3AUTH_NETWORK_TYPE = (typeof WEB3AUTH_NETWORK)[keyof typeof WEB3AUTH_NETWORK];
export declare const WEB3AUTH_NETWORK: {
readonly MAINNET: "mainnet";
readonly TESTNET: "testnet";
readonly CYAN: "cyan";
readonly AQUA: "aqua";
readonly CELESTE: "celeste";
readonly SAPPHIRE_DEVNET: "sapphire_devnet";
readonly SAPPHIRE_MAINNET: "sapphire_mainnet";
};
Adding a Custom Chain Configuration
chainConfig
const chainConfig = {
chainId: "0x1", // Please use 0x1 for Mainnet
rpcTarget: "https://rpc.ethereum.org",
displayName: "Ethereum Mainnet",
blockExplorerUrl: "https://etherscan.io/",
ticker: "ETH",
tickerName: "Ethereum",
logo: "https://images.toruswallet.io/eth.svg",
};
- Table
- Type Declarations
Parameter | Description |
---|---|
chainNamespace | Namespace of the chain |
chainId | Chain ID of the chain |
rpcTarget | RPC target URL for the chain |
wsTarget? | Web socket target URL for the chain |
displayName? | Display name for the chain |
blockExplorerUrl? | URL of the block explorer |
ticker? | Default currency ticker of the network |
tickerName? | Name for currency ticker |
decimals? | Number of decimals for the currency |
logo? | Logo for the token |
isTestnet? | Whether the network is testnet or not |
export declare const CHAIN_NAMESPACES: {
readonly EIP155: "eip155";
readonly SOLANA: "solana";
readonly CASPER: "casper";
readonly XRPL: "xrpl";
readonly OTHER: "other";
};
export type ChainNamespaceType = (typeof CHAIN_NAMESPACES)[keyof typeof CHAIN_NAMESPACES];
export type CustomChainConfig = {
chainNamespace: ChainNamespaceType;
/**
* The chain id of the chain
*/
chainId: string;
/**
* RPC target Url for the chain
*/
rpcTarget: string;
/**
* web socket target Url for the chain
*/
wsTarget?: string;
/**
* Display Name for the chain
*/
displayName?: string;
/**
* Url of the block explorer
*/
blockExplorerUrl?: string;
/**
* Default currency ticker of the network (e.g: ETH)
*/
ticker?: string;
/**
* Name for currency ticker (e.g: `Ethereum`)
*/
tickerName?: string;
/**
* Number of decimals for the currency ticker (e.g: 18)
*/
decimals?: number;
/**
* Logo for the token
*/
logo?: string;
/**
* Whether the network is testnet or not
*/
isTestnet?: boolean;
};
It's mandatory to pass the logo
parameter when using WalletServices.
Get the Chain Config for your preferred Blockchain from the Connect Blockchain Reference.
Adding a Private Key Provider
privateKeyProvider
privateKeyProvider
parameter helps you to connect with various wallet SDKs. These are
preconfigured RPC clients for different blockchains used to interact with the respective blockchain
networks.
It's mandatory to provide privateKeyProvider
for your corresponding chain namespace. To know more
in-depth about providers, have a look at the Providers reference.
You can choose between the following providers based on your usecase.
- EIP1193 Private Key Provider for EVM Compatible Chains
- Solana Private Key Provider for Solana Blockchain
- XRPL Private Key Provider for XRPL Blockchain
- Common Private Key Provider for Connecting to any Blockchain
- EIP1193 Provider
- Solana Provider
- XRPL Provider
- Common Provider
import { EthereumPrivateKeyProvider } from "@web3auth/ethereum-provider";
const privateKeyProvider = new EthereumPrivateKeyProvider({
config: { chainConfig },
});
const web3auth = new Web3AuthNoModal({
clientId: "", // Get your Client ID from the Web3Auth Dashboard
web3AuthNetwork: WEB3AUTH_NETWORK.SAPPHIRE_MAINNET,
privateKeyProvider,
});
import { SolanaPrivateKeyProvider } from "@web3auth/solana-provider";
const privateKeyProvider = new SolanaPrivateKeyProvider({
config: { chainConfig },
});
const web3auth = new Web3AuthNoModal({
clientId: "", // Get your Client ID from the Web3Auth Dashboard
web3AuthNetwork: WEB3AUTH_NETWORK.SAPPHIRE_MAINNET,
privateKeyProvider,
});
import { XrplPrivateKeyProvider } from "@web3auth/xrpl-provider";
const privateKeyProvider = new XrplPrivateKeyProvider({
config: { chainConfig },
});
const web3auth = new Web3AuthNoModal({
clientId: "", // Get your Client ID from the Web3Auth Dashboard
web3AuthNetwork: WEB3AUTH_NETWORK.SAPPHIRE_MAINNET,
privateKeyProvider,
});
import { CommonPrivateKeyProvider } from "@web3auth/base-provider";
const privateKeyProvider = new CommonPrivateKeyProvider({
config: { chainConfig },
});
const web3auth = new Web3AuthNoModal({
clientId: "", // Get your Client ID from the Web3Auth Dashboard
web3AuthNetwork: WEB3AUTH_NETWORK.SAPPHIRE_MAINNET,
privateKeyProvider,
});
Whitelabeling
Within the uiConfig
parameter, you can configure the Web3Auth NoModal according to your
application's requirements. It accepts a WhiteLabelData
object.
This is just one of the aspects of whitelabeling you can achieve with Web3Auth. To know more in-depth about how you can Whitelabel your application with Web3Auth Plug and Play Modal SDK, have a look at our Whitelabeling SDK Reference.
WhiteLabelData
- Table
- Interface
Parameter | Description |
---|---|
appName | App name to display in the UI |
appUrl | App url |
logoLight | App logo to use in light mode |
logoDark | App logo to use in dark mode |
defaultLanguage | language which will be used by web3auth. app will use browser language if not specified. if language is not supported it will use "en" |
mode | theme |
useLogoLoader | Use logo loader |
theme | Used to customize your theme |
tncLink | Language specific link for terms and conditions on torus-website. See (examples/vue-app) to configure |
privacyPolicy | Language specific link for privacy policy on torus-website. See (examples/vue-app) to configure |
export type WhiteLabelData = {
/**
* App name to display in the UI
*/
appName?: string;
/**
* App url
*/
appUrl?: string;
/**
* App logo to use in light mode
*/
logoLight?: string;
/**
* App logo to use in dark mode
*/
logoDark?: string;
/**
* language which will be used by web3auth. app will use browser language if not specified. if language is not supported it will use "en"
* en: english
* de: german
* ja: japanese
* ko: korean
* zh: mandarin
* es: spanish
* fr: french
* pt: portuguese
* nl: dutch
* tr: turkish
*
* @defaultValue en
*/
defaultLanguage?: LANGUAGE_TYPE;
/**
theme
*
* @defaultValue light
*/
mode?: THEME_MODE_TYPE;
/**
* Use logo loader
*
* @defaultValue false
*/
useLogoLoader?: boolean;
/**
* Used to customize your theme
*/
theme?: WHITE_LABEL_THEME;
/**
* Language specific link for terms and conditions on torus-website. See (examples/vue-app) to configure
* e.g.
* tncLink: {
* en: "http://example.com/tnc/en",
* ja: "http://example.com/tnc/ja",
* }
*/
tncLink?: Partial<Record<LANGUAGE_TYPE, string>>;
/**
* Language specific link for privacy policy on torus-website. See (examples/vue-app) to configure
* e.g.
* privacyPolicy: {
* en: "http://example.com/tnc/en",
* ja: "http://example.com/tnc/ja",
* }
*/
privacyPolicy?: Partial<Record<LANGUAGE_TYPE, string>>;
};
export declare const LANGUAGES: {
readonly en: "en";
readonly ja: "ja";
readonly ko: "ko";
readonly de: "de";
readonly zh: "zh";
readonly es: "es";
readonly fr: "fr";
readonly pt: "pt";
readonly nl: "nl";
readonly tr: "tr";
};
export type LANGUAGE_TYPE = (typeof LANGUAGES)[keyof typeof LANGUAGES];
export declare const THEME_MODES: {
readonly light: "light";
readonly dark: "dark";
readonly auto: "auto";
};
export type THEME_MODE_TYPE = (typeof THEME_MODES)[keyof typeof THEME_MODES];
export type WHITE_LABEL_THEME = {
/**
* `primary` color that represents your brand
* Will be applied to elements such as primary button, nav tab(selected), loader, input focus, etc.
*/
primary?: string;
/**
* `onPrimary` color that is meant to contrast with the primary color
* Applies to elements such as the text in a primary button or nav tab(selected), blocks of text on top of a primary background, etc.
*/
onPrimary?: string;
};
uiConfig: {
appName: "W3A Heroes",
appUrl: "https://web3auth.io",
logoLight: "https://web3auth.io/logo-light.png",
logoDark: "https://web3auth.io/logo-dark.png",
defaultLanguage: "en",
mode: "theme",
useLogoLoader: true,
},
Returns
Object
: The web3auth instance with all its methods and events.
Configuring Adapters
An adapter in Web3Auth is a pluggable package that implements the IAdapter
interface. Some, like
AuthAdapter
, extend the BaseAdapter
class, which also implements IAdapter
. Adapters can be
plugged into or removed from the Web3Auth instance. After successful user login, each adapter
exposes a provider to invoke RPC calls on the wallet and connected blockchain. You can configure and
use any Web3Auth adapter when initializing Web3AuthNoModal.
Configuring Auth Adapter [Social Logins]
The default adapter of Web3Auth is the auth-adapter
. This adapter is
a wrapper around the auth
library from Web3Auth and enables the
social login features. For customising features of the main Web3Auth flow, like
Whitelabel, Custom Authentication, etc.
you need to customise the Auth Adapter.
Checkout the auth-adapter
SDK Reference for more details on
different configurations you can pass for customisations.
Whitelabeling
whiteLabel
For customising the redirect screens while logging in and constructing the key, you need to pass on
whiteLabel
configurations to the adapterSettings
property of the
auth-adapter
.
This is just one of the aspects of whitelabeling you can achieve with Web3Auth. To know more in depth about how you can Whitelabel your application with Web3Auth, have a look at our Whitelabeling SDK Reference.
import { AuthAdapter, WHITE_LABEL_THEME, WhiteLabelData } from "@web3auth/auth-adapter";
import {
CHAIN_NAMESPACES,
IProvider,
UX_MODE,
WALLET_ADAPTERS,
WEB3AUTH_NETWORK,
} from "@web3auth/base";
const authAdapter = new AuthAdapter({
adapterSettings: {
clientId, //Optional - Provide only if you haven't provided it in the Web3Auth Instantiation Code
network: WEB3AUTH_NETWORK.SAPPHIRE_MAINNET, // Optional - Provide only if you haven't provided it in the Web3Auth Instantiation Code
uxMode: UX_MODE.REDIRECT,
whiteLabel: {
appName: "W3A Heroes",
appUrl: "https://web3auth.io",
logoLight: "https://web3auth.io/images/web3auth-logo.svg",
logoDark: "https://web3auth.io/images/web3auth-logo---Dark.svg",
defaultLanguage: "en", // en, de, ja, ko, zh, es, fr, pt, nl, tr
mode: "dark", // whether to enable dark mode. defaultValue: auto
theme: {
primary: "#00D1B2",
} as WHITE_LABEL_THEME,
useLogoLoader: true,
} as WhiteLabelData,
},
privateKeyProvider,
});
web3auth.configureAdapter(authAdapter);
Multi Factor Authentication
mfaLevel
For a dApp, we provide various options to set up Multi-Factor Authentication. You can customize the
MFA screen by setting the mfaLevel
argument. You can enable or disable a backup factor and change
their order. Currently, there are four values for mfaLevel
:
default
: presents the MFA screen every third loginoptional
: presents the MFA screen on every login, but you can skip itmandatory
: make it mandatory to set up MFA after loginnone
: skips the MFA setup screen
import { AuthAdapter } from "@web3auth/auth-adapter";
const authAdapter = new AuthAdapter({
loginSettings: {
mfaLevel: "mandatory", // default, optional, mandatory, none
},
});
Users of default Web3Auth verifiers may see MFA prompts across all dApps using these verifiers if they've enabled MFA on any one of them. This MFA can't be turned off once activated.
mfaSettings
For customising the MFA settings, you need to pass on mfaSettings
configurations to the
adapterSettings
property of the AuthAdapter
.
Read more about mfaSettings
in the Multi Factor Authentication Section SDK Reference.
Note: mfaSettings
is available for SCALE and above plan users.
import { AuthAdapter } from "@web3auth/auth-adapter";
const authAdapter = new AuthAdapter({
adapterSettings: {
// SCALE and above plan only feature
mfaSettings: {
deviceShareFactor: {
enable: true,
priority: 1,
mandatory: true, // at least two factors are mandatory
},
backUpShareFactor: {
enable: true,
priority: 2,
mandatory: true, // at least two factors are mandatory
},
socialBackupFactor: {
enable: true,
priority: 3,
mandatory: false,
},
passwordFactor: {
enable: true,
priority: 4,
mandatory: false,
},
passkeysFactor: {
enable: true,
priority: 5,
mandatory: false,
},
authenticatorFactor: {
enable: true,
priority: 6,
mandatory: false,
},
},
},
});
Custom Authentication
loginConfig
With Web3Auth, you have the option to configure logins using your own authentication services. For adding your own authentication, you have to first configure your verifiers in the Web3Auth Dashboard.
Custom Authentication in Web3Auth is supported by the Auth Adapter, which is the default adapter for
the Web3Auth SDK. For this, you need to configure the loginConfig
parameter in the
adapterSettings
of the auth-adapter
package.
Refer to the Custom Authentication Documentation for more information.
Example
Since we're using the @web3auth/no-modal
, ie. the Plug and Play No Modal SDK, the loginConfig
can include custom JWT-based authentication as well. This way, we can use any of our preferred login
providers and further setup their configs while logging the user in and passing over the
extraLoginOptions
in the connectTo
function. Read more about this in the
Custom Authentication Guide.
import { AuthAdapter } from "@web3auth/auth-adapter";
const authAdapter = new AuthAdapter({
adapterSettings: {
loginConfig: {
jwt: {
verifier: "YOUR-VERIFIER-NAME-ON-WEB3AUTH-DASHBOARD",
typeOfLogin: "jwt",
clientId: "YOUR-CLIENTID-FROM-LOGIN-PROVIDER",
},
},
},
privateKeyProvider,
});
web3auth.configureAdapter(authAdapter);
Configuring External Wallet Adapters
External Adapters helps you to connect with external wallets like Metamask, Coinbase, Phantom, and
more. To configure an adapter, create the instance of the adapter and pass the instance in the
configureAdapter
function.
Default EVM Adapter
The @web3auth/default-evm-adapter package enables seamless detection of injected EVM wallets and WalletConnect-supported wallets, allowing interaction with just a single line of code. Learn more about Default EVM Adapter.
Default Solana Adapter
The @web3auth/default-solana-adapter enables seamless detection of injected Solana wallets, allowing interaction with just a single line of code. Learn more about Default Solana Adapter.
Refer to the Adapters documentation to know more deeply about what adapters are available and how to configure them.
Configuring Plugins
This step is optional. If you do not plan to use any plugins, you can skip this section.
Plugins are essentially extensions to the core functionality of Web3Auth, allowing you to add additional features to your dApp. These features can be used to extend the UI functionalities, making your integration more interoperable, and a lot more, even having the functionality to be customised extremely and to your liking.
Refer to the Wallet Services Plugin Documentation to know more deeply about how you utilise our plugins to extend the functionality of your dApp.
import { WalletServicesPlugin } from "@web3auth/wallet-services-plugin";
const walletServicesPlugin = new WalletServicesPlugin();
web3auth.addPlugin(walletServicesPlugin); // Add the plugin to web3auth
Initializing Web3Auth
init()
The final step in the whole initialization process is the initialize the Web3AuthNoModal
instance,
ie. web3auth
in our case.
This is done by calling the init
function of the web3auth
instance we created above.
await web3auth.init();
This is a simple function, that doesn't take any input, nor does return anything. It just makes sure
that the web3auth
instance is initialized and ready for the user to log in.
The Web3Auth No Modal React Hooks SDK doesn't require an additional step to init from v9 onwards, it's auto-inited.
Quick Starts
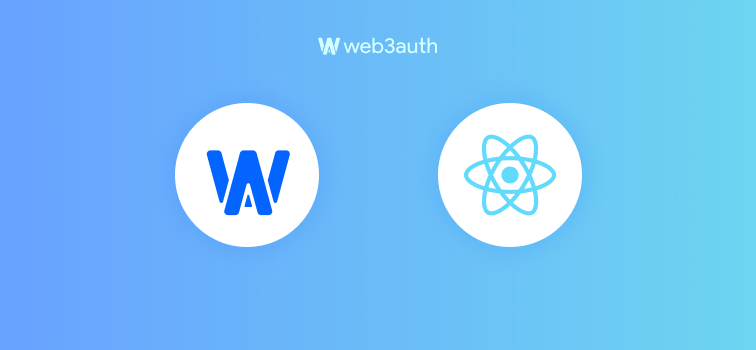
PnP No Modal SDK React Quick Start
A quick integration of Plug and Play No Modal SDK in React
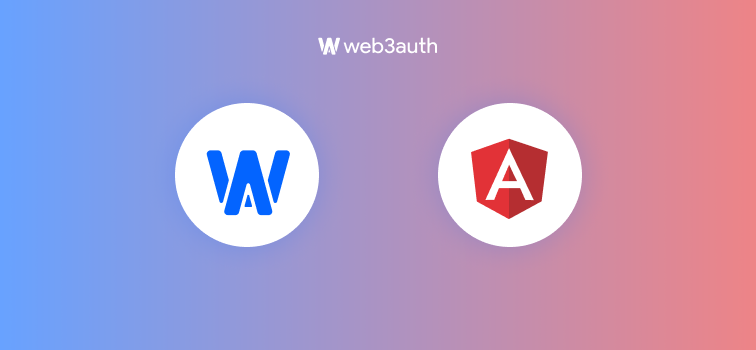
PnP No Modal SDK Angular Quick Start
A quick integration of Plug and Play No Modal SDK in angular
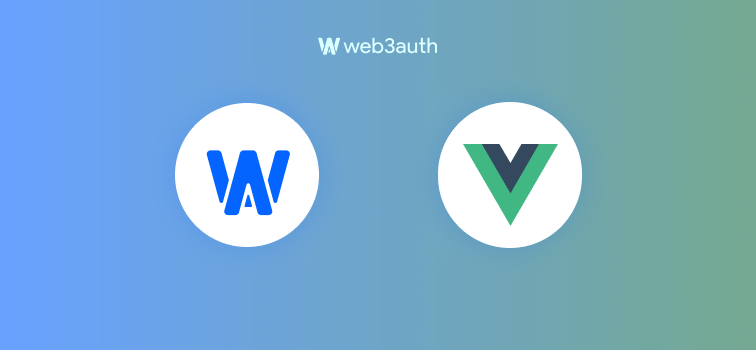
PnP No Modal SDK Vue Quick Start
A quick integration of Plug and Play No Modal SDK in Vue
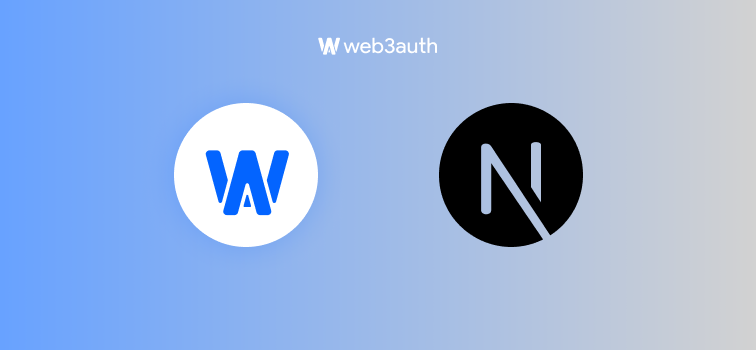
PnP No Modal SDK NextJS Quick Start
A quick integration of Plug and Play No Modal SDK in NextJS
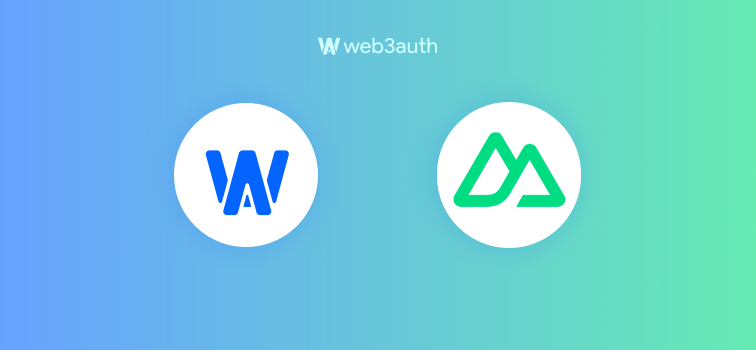
PnP No Modal SDK Nuxt Quick Start
A quick integration of Plug and Play No Modal SDK in Nuxt
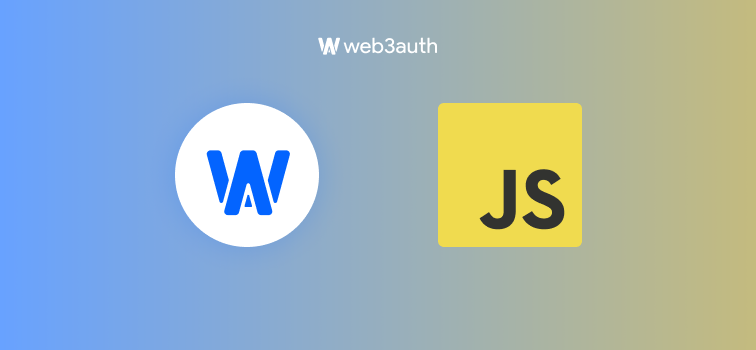
PnP No Modal SDK Vanilla JS Quick Start
A quick integration of Plug and Play No Modal SDK in Vanilla JS