Wagmi Connector for Web3Auth PnP Web SDKs
@web3auth/web3auth-wagmi-connector
wagmi is a collection of React Hooks containing everything you need to start working with
Ethereum. @web3auth/web3auth-wagmi-connector
is a connector for the popular
wagmi library to help you integrate web3auth plug and play packages.
It works with both the @web3auth/no-modal
as well as the @web3auth/modal
packages.
This package can be used to initialize a wagmi client that will seamlessly manage the interaction(wallet connection state and configuration, such as: auto-connection, connectors, and ethers providers) of your dApp with Web3Auth.
Checkout the Modal Example Apps to see how wagmi works with Web3Auth.
This version of wagmi connector is made for @wagmi/core
v2.6.5
.
Installation
- npm
- Yarn
- pnpm
npm install --save @web3auth/web3auth-wagmi-connector
yarn add @web3auth/web3auth-wagmi-connector
pnpm add @web3auth/web3auth-wagmi-connector
Initialization
Import the Web3AuthConnector
class from @web3auth/web3auth-wagmi-connector
import { Web3AuthConnector } from "@web3auth/web3auth-wagmi-connector";
Arguments
Web3AuthConnectorParams
- Table
- Interface
Parameter | Description |
---|---|
web3AuthInstance | Pass the Web3Auth instance here. It's the mandatory field and accepts IWeb3Auth or IWeb3AuthModal . |
loginParams? | Login Parameters (only meant while using the @web3auth/no-modal package). It accepts OpenloginLoginParams . |
modalConfig? | Initialisation Parameters (only meant while using the @web3auth/modal package). It accepts Record<WALLET_ADAPTER_TYPE, ModalConfig> . |
export interface Web3AuthConnectorParams {
web3AuthInstance: IWeb3Auth | IWeb3AuthModal;
loginParams?: OpenloginLoginParams;
modalConfig?: Record<WALLET_ADAPTER_TYPE, ModalConfig>;
}
modalConfig
- Table
- Interface
modalConfig: { ADAPTER : { params } }
Parameter | Description |
---|---|
label | Label of the adapter you want to configure. It's a mandatory field which accepts string . |
showOnModal? | Whether to show an adapter in modal or not. Default value is true . |
showOnDesktop? | Whether to show an adapter in desktop or not. Default value is true . |
showOnMobile? | Whether to show an adapter in mobile or not. Default value is true . |
Additionally, to configure the Auth Adapter's each login method, we have the loginMethods?
parameter.
Parameter | Description |
---|---|
loginMethods? | To configure visibility of each social login method for the auth adapter. It accepts LoginMethodConfig as a value. |
initModal(params?: {
modalConfig?: Record<WALLET_ADAPTER_TYPE, ModalConfig>;
}): Promise<void>;
interface ModalConfig extends BaseAdapterConfig {
loginMethods?: LoginMethodConfig;
}
interface BaseAdapterConfig {
label: string;
showOnModal?: boolean;
showOnMobile?: boolean;
showOnDesktop?: boolean;
}
loginMethods: { label: { params } }
In loginMethods
, you can configure the visibility of each social login method for the auth
adapter. The social login is corresponded by the label
parameter. Below is the table indicating
the different params
available for customization.
For labels
you can choose between these options: google
, facebook
, twitter
, reddit
,
discord
, twitch
, apple
, line
, github
, kakao
, linkedin
, weibo
, wechat
,
email_passwordless
- Table
- Type Declaration
params
Parameter | Description |
---|---|
name? | Display Name. It accepts string as a value. |
description? | Description for the button. If provided, it renders as a full length button. else, icon button. It accepts string as a value. |
logoHover? | Logo to be shown on mouse hover. It accepts string as a value. |
logoLight? | Light logo for dark background. It accepts string as a value. |
logoDark? | Dark logo for light background. It accepts string as a value. |
mainOption? | Show login button on the main list. It accepts oolean as a value. Default value is false. |
showOnModal? | Whether to show the login button on modal or not. Default value is true. |
showOnDesktop? | Whether to show the login button on desktop. Default value is true. |
showOnMobile? | Whether to show the login button on mobile. Default value is true. |
declare type LoginMethodConfig = Record<
string,
{
/**
* Display Name. If not provided, we use the default for openlogin app
*/
name: string;
/**
* Description for button. If provided, it renders as a full length button. else, icon button
*/
description?: string;
/**
* Logo to be shown on mouse hover. If not provided, we use the default for openlogin app
*/
logoHover?: string;
/**
* Logo to be shown on dark background (dark theme). If not provided, we use the default for openlogin app
*/
logoLight?: string;
/**
* Logo to be shown on light background (light theme). If not provided, we use the default for openlogin app
*/
logoDark?: string;
/**
* Show login button on the main list
*/
mainOption?: boolean;
/**
* Whether to show the login button on modal or not
*/
showOnModal?: boolean;
/**
* Whether to show the login button on desktop
*/
showOnDesktop?: boolean;
/**
* Whether to show the login button on mobile
*/
showOnMobile?: boolean;
}
>;
Usage
Since this package acts like a connector, it basically takes in your whole Web3Auth instance and makes it readable for the wagmi library. While connecting the web3auth web packages, you need to initialize the Web3Auth Instance as mentioned in the modal docs and no-modal docs. You can configure this instance with all the options available in Web3Auth Modal package and set it up as you wish.
Modal SDK
While all the parameters can be passed directly to the instance, the only parameters that remain
during the initialisation remains (passed on to the initModal()
function). You can pass these
parameters to the modalConfig
object in the Web3AuthConnector
class.
modalConfig: {
[WALLET_ADAPTERS.AUTH]: {
loginMethods: {
google: {
name: "google login",
logoDark: "url to your custom logo which will shown in dark mode",
},
facebook: {...},
},
},
}
Example
No Modal SDK
While all the parameters can be passed directly to the instance, the only parameters that remain
during the login remains (passed on to the connectTo()
function). You can pass these parameters to
the loginParams
object in the Web3AuthConnector
class.
It is mandatory to pass loginParams
object while using the connector with Web3Auth Core package.
This is because the connectTo()
function requires params to connect to the adapter/ social login
desired by the user.
- JWT
- Auth0
- Discord
- Twitch
- Apple
- GitHub
- Line
- Email Passwordless
- SMS Passwordless
loginParams: {
loginProvider: 'google',
}
loginParams: {
loginProvider: 'facebook',
}
loginParams: {
loginProvider: "jwt",
extraLoginOptions: {
id_token: "idToken", // in JWT Format
verifierIdField: "sub", // same as your JWT Verifier ID
}
}
loginParams: {
loginProvider: "jwt",
extraLoginOptions: {
verifierIdField: "sub", // same as your JWT Verifier ID
domain: "https://YOUR-APPLICATION-DOMAIN", // your Auth0 domain
},
}
loginParams: {
loginProvider: 'email_passwordless',
extraLoginOptions: {
login_hint: "hello@web3auth.io", // email to send the OTP to
},
}
loginParams: {
loginProvider: 'sms_passwordless',
extraLoginOptions: {
login_hint: "+65-XXXXXXX",
}
}
loginParams: {
loginProvider: 'reddit',
}
loginParams: {
loginProvider: 'discord',
}
loginParams: {
loginProvider: 'twitch',
}
loginParams: {
loginProvider: 'apple',
}
loginParams: {
loginProvider: 'github',
}
loginParams: {
loginProvider: 'linkedin',
}
loginParams: {
loginProvider: 'twitter',
}
loginParams: {
loginProvider: 'weibo',
}
loginParams: {
loginProvider: 'line',
}
Examples
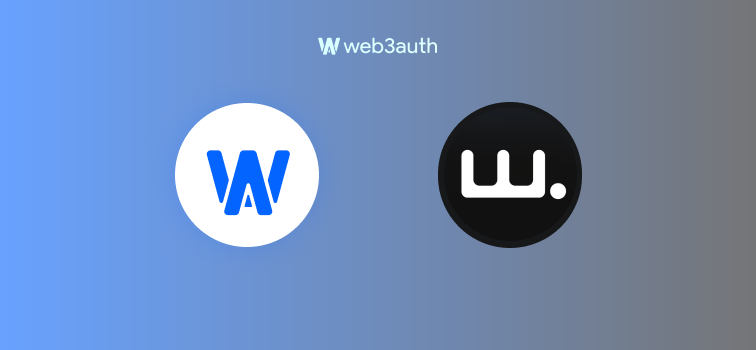
Using PnP Modal SDK with WAGMI
Using Plug and Play Modal SDK with WAGMI Hooks
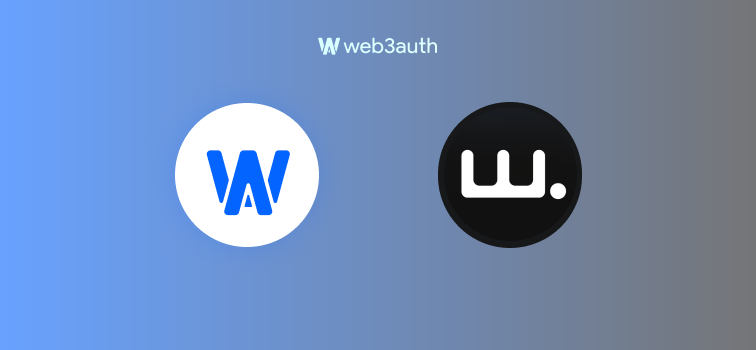
Using PnP No Modal SDK with WAGMI
Using Plug and Play No Modal SDK with WAGMI Hooks